An Interest In:
Web News this Week
- April 16, 2024
- April 15, 2024
- April 14, 2024
- April 13, 2024
- April 12, 2024
- April 11, 2024
- April 10, 2024
Map,Reduce,For-each Loops in js
Reduce:
The reduce() function iterates over the elements of an array and accumulates a single result by applying a provided function against an accumulator and each element in the array.
const numbers = [1, 2, 3, 4, 5];const sum = numbers.reduce((accumulator, currentValue) => accumulator + currentValue, 0);console.log(sum); // Output: 15
Industry Scenario: Consider an e-commerce platform where you need to calculate the total price of items in a shopping cart. You have an array of objects representing each item with properties like price and quantity. You can use reduce() to sum up the total price:
const cartItems = [ { name: 'Shirt', price: 20, quantity: 2 }, { name: 'Pants', price: 30, quantity: 1 }, { name: 'Shoes', price: 50, quantity: 1 }];const totalPrice = cartItems.reduce((total, item) => total + (item.price * item.quantity), 0);console.log(totalPrice); // Output: 120
ForEach:
The forEach() function executes a provided function once for each array element.
const numbers = [1, 2, 3, 4, 5];numbers.forEach(number => console.log(number));// Output:// 1// 2// 3// 4// 5
Industry Scenario: Consider a scenario in a project management tool where you want to update the status of each task in a list. You can use forEach() to iterate through the array of tasks and update their status:
const tasks = [ { id: 1, name: 'Task 1', status: 'Pending' }, { id: 2, name: 'Task 2', status: 'InProgress' }, { id: 3, name: 'Task 3', status: 'Pending' }];tasks.forEach(task => { if (/* condition for updating status */) { task.status = 'Completed'; }});
reduce()
const cartItems = [ { name: 'Shirt', price: 20, quantity: 2 }, { name: 'Pants', price: 30, quantity: 1 }, { name: 'Shoes', price: 50, quantity: 1 }];const totalPrice = cartItems.reduce((total, item) => total + (item.price * item.quantity), 0);console.log(totalPrice); // Output: 120
Original Link: https://dev.to/rohiitbagal/mapreducefor-each-loops-in-js-1e2p

Dev To
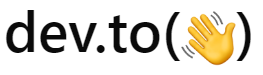
More About this Source Visit Dev To