An Interest In:
Web News this Week
- April 13, 2024
- April 12, 2024
- April 11, 2024
- April 10, 2024
- April 9, 2024
- April 8, 2024
- April 7, 2024
Day25 - A cheatsheet to get Fake/Mock Data in Python using Faker
You can use Python to come up with your company's name and slogan
We will be using the library Faker
Install the library
pip install faker
Now let's import the library and create an instance of "Faker"
from faker import Fakerfake = Faker()
Seeding
Seeding ensures that the same set of random data is returned during every run. This is very useful when you want to compare functions or models in the same environment without changes in the dataset.
from faker import FakerFaker.seed(999)fake = Faker()
Names
print(fake.name())'''Wesley Turner'''print(fake.first_name())'''Alexandra'''print(fake.last_name())'''Aguilar'''print(fake.name_male())'''Charles Hanna'''print(fake.name_female())'''Shannon Walker'''print(fake.name_nonbinary())'''Christopher Brennan'''
Address and Phone Numbers
from faker import FakerFaker.seed(999)fake = Faker()print(fake.address())'''987 Anderson UnionDawnville, ID 20051'''print(fake.phone_number())'''904-636-1403x832'''
But these all seem like American Names, Addresses, and Phone numbers. Let's get data for some other region.
Locale Data based on Region
We can set the region or localize the data by passing a string during instantiation. Not all locales support all the functions in Faker. You can find all the locales here
from faker import FakerFaker.seed(999)## Seting locale to India and language to hindifake = Faker('hi_IN')print(fake.name())''', '''print(fake.address())'''13/24 -140383'''print(fake.phone_number())'''+91 521 8975277'''
We set the locale to India and the language to Hindi. Let's try setting the locale to France and language to french.
from faker import FakerFaker.seed(999)fake = Faker('fr_FR')print(fake.name())'''Roland Baudry'''print(fake.address())'''25, rue de Gerard90463 Sanchez-la-Fort'''print(fake.phone_number())'''+33 (0)1 38 32 52 18'''
You can also pass in multiple locales as a list.
from faker import FakerFaker.seed(999)fake = Faker(['hi_IN','en_US','fr_FR'])for _ in range(5): print(fake.phone_number()) print("-----------------")'''+33 (0)5 99 87 72 51-----------------+1-324-904-6361x4038-----------------+91 252 1897527----------------------00031364850----------------------001-271-698-2331x03084----------------------'''
Fake Company Names and Catch Phrase
from faker import FakerFaker.seed(999)fake = Faker()for _ in range(5): print(f'{fake.company()} : {fake.catch_phrase()}') print("-x-x-")'''Miller, Brennan and Berry : Pre-emptive mission-critical capacity-x-x-Flores, Norton and Bruce : Cross-platform secondary core-x-x-Baker PLC : Business-focused tangible time-frame-x-x-Sanders-Bowers : Focused responsive initiative-x-x-Nelson and Sons : Stand-alone motivating database-x-x-'''
I believe all this actually fetched from some database, so this isn't like GPT-3 which actually generates a name.
Faking Profiles
from faker import FakerFaker.seed(999)fake = Faker(['en_IN'])print(f'{fake.profile()}')'''{'job': 'Cartographer', 'company': 'Mangat, Loke and Korpal', 'ssn': '251-32-4904', 'residence': '61/403, Chawla Circle, Bathinda-189752', 'current_location': (Decimal('87.4766035'), Decimal('170.848372')), 'blood_group': 'O-', 'website': ['http://www.bhalla.com/', 'https://gokhale.com/'], 'username': 'vrajagopal', 'name': 'Ivan Das', 'sex': 'F', 'address': 'H.No. 681, Mander Marg, Howrah-684709', 'mail': '[email protected]', 'birthdate': datetime.date(2019, 10, 8)}'''
Faking Emails
from faker import FakerFaker.seed(999)fake = Faker(['en_IN'])print(fake.email())'''[email protected]'''print(fake.safe_email() )'''[email protected]'''print(fake.free_email() )'''[email protected]'''print(fake.company_email() )'''[email protected]'''
Generating Fake Python Data Types
from faker import FakerFaker.seed(999)fake = Faker(['en_IN'])print(fake.pytuple())'''('https://vyas.com/posts/list/main/author.htm', 'nDKnTZlGdbkYAjJiUCWy', 2125, '[email protected]', 'ZLIvUSsViQwFPkfdaGhy', 7650, 'ZbBODSZctqOcGVcxOWIn')'''print(fake.pylist())'''['DqwQiBApBNXchzoOOsDB', 'uxrFsPxsaLVPzMouLrct', -3001403.6558895, '[email protected]', -783180386.336077, 'ODrlFCjaPMlWoEGrIvcY', '[email protected]']'''print(fake.pydict())'''{'hic': 'kSKSsguiUhAHdBwXsvPU', 'non': 'NnApyVhwOfvBBidAOfCa', 'sit': '[email protected]', 'cumque': 86753382.7739197, 'aut': 93.434062857687, 'voluptatem': 321.537771434202, 'dignissimos': 'xememaWRlowIFAtOAEMo', 'sint': 5095, 'vero': 1680, 'molestiae': Decimal('-934.685870829972'), 'reiciendis': 9133, 'quo': 'tRBgKmfGLRRdMFpEyPAq', 'fuga': '[email protected]'}'''print(fake.pyiterable())'''[datetime.datetime(1990, 2, 3, 3, 11, 17), 1446, 611903747.767127, 'http://www.sami.net/', 'iJIxPgnNWUsZztHjzhuc', 'JZufaRqcvQJujWAgbosd', Decimal('74468.5598767266'), '[email protected]']'''
Original Link: https://dev.to/rahulbanerjee99/day25-a-cheatsheet-to-get-fake-mock-data-in-python-using-faker-56ad

Dev To
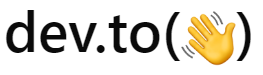
More About this Source Visit Dev To