An Interest In:
Web News this Week
- April 19, 2024
- April 18, 2024
- April 17, 2024
- April 16, 2024
- April 15, 2024
- April 14, 2024
- April 13, 2024
Next.js Authentication
Authentication is an important aspect of web development, ensuring that users can safely access protected resources and data in an application. The popular React Next.js framework provides developers with powerful tools for building secure recognition systems.
Understand Authentication
Authentication involves verifying a user's identity before accessing application resources. This process usually includes credentials such as usernames, passwords, tags, or biometric data. Implementing validation in a Next.js application requires careful consideration of security, user experience, and scalability.
Authentication Techniques
1. Session Based Authentication
- In session-based authentication, the server creates a session for each authenticated user, usually using cookies to maintain session state.
- Next.js provides built-in support for server-side authentication (SSR), making it seamless to implement session-based authentication using frameworks like Express.js or NextAuth.js.
- To implement session-based authentication in Next.js, you can use server-side middleware to verify session tokens and protect routes.
2. Token based authentication
- Token-based authentication involves issuing tokens to authenticated users that are included with each request to access protected resources.
- Next.js works with multiple token-based authentication schemes such as JSON Web Tokens (JWT) and OAuth.
- Implementing token-based authentication in Next.js requires client-side processing to store and manage secure tokens, as well as server-side authentication to verify token authenticity and permissions.
3. Third Party Verification
- Next.js applications may use third-party recognition providers such as Google, Facebook or GitHub to recognize users.
- NextAuth.js is a popular authentication library for Next.js that simplifies integration with third-party providers and provides a seamless authentication experience.
- Integrate third-party authentication in Next.js including configuring an OAuth or OpenID Connect (OIDC) provider and handling authentication calls in your application.
Perform validation in Next.js
1. install dependencies
- Depending on the authentication method you choose, set the required dependencies, such as next-auth for NextAuth.js or jsonwebtoken for JWT-based authentication.
2. Configure authentication
- Configure authentic authentication providers, configure session management settings, and define authentication routes and callbacks if needed.
// pages/api/auth/[...nextauth].jsimport NextAuth from 'next-auth';import Providers from 'next-auth/providers';export default NextAuth({ providers: [ Providers.Google({ clientId: process.env.GOOGLE_CLIENT_ID, clientSecret: process.env.GOOGLE_CLIENT_SECRET, }), // Add other providers as needed ], // Optional SQL or MongoDB database to persist users database: process.env.DATABASE_URL,});
- Configure client-side retention mechanisms for bookmarks or session data, ensuring security and compatibility across browsers.
3. Protect Route
- Use middleware or traffic guards to protect routes that require authentication. Redirect unauthenticated users to the login page or display an appropriate error message.
// components/RequireAuth.jsimport { useSession } from 'next-auth/react';const RequireAuth = ({ children }) => { const { data: session, status } = useSession(); if (status === 'loading') { return <div>Loading...</div>; } if (!session) { // Redirect to login page if user is not authenticated return <Redirect to="/login" />; } return children;};export default RequireAuth;
4. Handle Authentication Events
- Implement logic to handle authentication events such as login, logout, or token expiration.
- Dynamically update UI components based on recognition status, providing user experience.
// pages/protected.jsimport RequireAuth from '../components/RequireAuth';const ProtectedPage = () => { return ( <RequireAuth> <div> <h1>Protected Page</h1> <p>This page is protected. Only authenticated users can access it.</p> </div> </RequireAuth> );};export default ProtectedPage;
Best practices for introducing Next.js
- Use HTTPS: Make sure your application uses HTTPS to encrypt data sent between client and server to prevent man-in-the-middle attacks.
- Secure Session Management:: Implement secure session management practices such as using the HttpOnly and Secure flags for cookies and implementing a strong session termination policy.
- Sanitize user input: Protect your application from common security vulnerabilities such as cross-site scripting (XSS) and SQL injection by sanitizing user input and using parameterized queries.
The results
Next.js gives developers the flexibility and tools they need to implement secure authentication systems in their applications. By understanding the different authentication methods and following best practices, you can create a secure and user-friendly authentication experience that protects your users' data and privacy. Whether you choose session-based authentication, token-based authentication, or third-party authentication, Next.js offers the flexibility to efficiently meet your authentication requirements.
Original Link: https://dev.to/syedmuhammadaliraza/nextjs-authentication-3oc7

Dev To
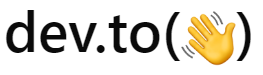
More About this Source Visit Dev To