An Interest In:
Web News this Week
- April 18, 2024
- April 17, 2024
- April 16, 2024
- April 15, 2024
- April 14, 2024
- April 13, 2024
- April 12, 2024
April 18, 2024 04:09 am GMT
Original Link: https://dev.to/hyperkai/min-max-argmin-and-argmax-in-pytorch-2d6
min(), max(), argmin() and argmax() in PyTorch
*Memos:
- My post explains aminmax(), amin() and amax().
- My post explains minimum(), maximum(), kthvalue() and topk().
min() can get the one or more minimum values of a 0D or more D tensor as shown below:
*Memos:
min()
can be called both from torch and a tensor.- Setting a dimension(
dim
) to the 2nd argument withtorch
or the 1st argument with a tensor gets zero or more 1st minimum values and the indices of them. - Only zero or more integers, floating-point numbers or boolean values can be used so zero or more complex numbers cannot be used.
import torchmy_tensor = torch.tensor([[5, 4, 7, 7], [6, 5, 3, 5], [3, 8, 9, 3]])torch.min(my_tensor)my_tensor.min()# tensor(3)torch.min(my_tensor, 0)my_tensor.min(0)torch.min(my_tensor, -2)my_tensor.min(-2)# torch.return_types.min(# values=tensor([3, 4, 3, 3]),# indices=tensor([2, 0, 1, 2]))torch.min(my_tensor, 1)my_tensor.min(1)torch.min(my_tensor, -1)my_tensor.min(-1)# torch.return_types.min(# values=tensor([4, 3, 3]),# indices=tensor([1, 2, 0]))my_tensor = torch.tensor([[5., 4., 7., 7.], [6., True, 3., 5.], [3., 8., False, 3.]])torch.min(my_tensor, 0)my_tensor.min(0)torch.min(my_tensor, -2)my_tensor.min(-2)# torch.return_types.min(# values=tensor([3., 1., 0., 3.]),# indices=tensor([2, 1, 2, 2]))
max() can get the one or more maximum values of a 0D or more D tensor as shown below:
*Memos:
max()
can be called both fromtorch
and a tensor.- Setting a dimension(
dim
) to the 2nd argument withtorch
or the 1st argument with a tensor gets zero or more 1st maximum values and the indices of them. - Only zero or more integers, floating-point numbers or boolean values can be used so zero or more complex numbers cannot be used.
import torchmy_tensor = torch.tensor([[5, 4, 7, 7], [6, 5, 3, 5], [3, 8, 9, 3]])torch.max(my_tensor)my_tensor.max()# tensor(9)torch.max(my_tensor, 0)my_tensor.max(0)torch.max(my_tensor, -2)my_tensor.max(-2)# torch.return_types.max(# values=tensor([6, 8, 9, 7]),# indices=tensor([1, 2, 2, 0]))torch.max(my_tensor, 1)my_tensor.max(1)torch.max(my_tensor, -1)my_tensor.max(-1)# torch.return_types.max(# values=tensor([7, 6, 9]),# indices=tensor([2, 0, 2]))my_tensor = torch.tensor([[5., 4., 7., 7.], [6., True, 3., 5.], [3., 8., False, 3.]])torch.max(my_tensor, 0)my_tensor.max(0)torch.max(my_tensor, -2)my_tensor.max(-2)# torch.return_types.max(# values=tensor([6., 8., 7., 7.]),# indices=tensor([1, 2, 0, 0]))
argmin() can get the indices of the 1st minimum values of a 0D or more D tensor as shown below:
*Memos:
argmin()
can be called both fromtorch
and a tensor.- The 2nd argument with
torch
or the 1st argument with a tensor is a dimension(dim
). - Zero or more integers, floating-point numbers or boolean values can be used so zero or more complex numbers cannot be used except the 1D tensor of a complex number with
dim=0
ordim=-1
.
import torchmy_tensor = torch.tensor([[5, 4, 7, 7], [6, 5, 3, 5], [3, 8, 9, 3]])torch.argmin(my_tensor)my_tensor.argmin()# tensor(6)torch.argmin(my_tensor, 0)my_tensor.argmin(0)torch.argmin(my_tensor, -2)my_tensor.argmin(-2)# tensor([2, 0, 1, 2])torch.argmin(my_tensor, 1)my_tensor.argmin(1) torch.argmin(my_tensor, -1)my_tensor.argmin(-1) # tensor([1, 2, 0])my_tensor = torch.tensor([[5., 4., 7., 7.], [6., True, 3., 5.], [3., 8., False, 3.]])torch.argmin(my_tensor, 0)my_tensor.argmin(0)torch.argmin(my_tensor, -2)my_tensor.argmin(-2)# tensor([2, 1, 2, 2])my_tensor = torch.tensor([5+7j])torch.argmin(my_tensor, 0)my_tensor.argmin(0)torch.argmin(my_tensor, -1)my_tensor.argmin(-1)# tensor(0)
argmax() can get the indices of the 1st maximum values of a 0D or more D tensor:
*Memos:
argmax()
can be called both fromtorch
and a tensor.- The 2nd argument with
torch
or the 1st argument with a tensor is a dimension(dim
). - Zero or more integers, floating-point numbers or boolean values can be used so zero or more complex numbers cannot be used except the 1D tensor of a complex number with
dim=0
ordim=-1
.
import torchmy_tensor = torch.tensor([[5, 4, 7, 7], [6, 5, 3, 5], [3, 8, 9, 3]])torch.argmax(my_tensor)my_tensor.argmax()# tensor(10)torch.argmax(my_tensor, 0)my_tensor.argmax(0)torch.argmax(my_tensor, -2)my_tensor.argmax(-2)# tensor([1, 2, 2, 0])torch.argmax(my_tensor, 1)my_tensor.argmax(1)torch.argmax(my_tensor, -1)my_tensor.argmax(-1)# tensor([2, 0, 2])my_tensor = torch.tensor([[5., 4., 7., 7.], [6., True, 3., 5.], [3., 8., False, 3.]])torch.argmax(my_tensor, 0)my_tensor.argmax(0)torch.argmax(my_tensor, -2)my_tensor.argmax(-2)# tensor([1, 2, 0, 0])my_tensor = torch.tensor([5+7j])torch.argmax(my_tensor, 0)my_tensor.argmax(0)torch.argmax(my_tensor, -1)my_tensor.argmax(-1)# tensor(0)
Original Link: https://dev.to/hyperkai/min-max-argmin-and-argmax-in-pytorch-2d6
Share this article:
Tweet
View Full Article

Dev To
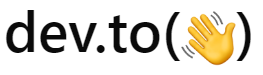
More About this Source Visit Dev To