An Interest In:
Web News this Week
- April 16, 2024
- April 15, 2024
- April 14, 2024
- April 13, 2024
- April 12, 2024
- April 11, 2024
- April 10, 2024
The Dark Modal: Crafting Reusable React Modals
Introduction: Conjuring the Dark Modal
In the realm of React development, modals are akin to mystical portals, providing dynamic overlays that can transport users to different contextual experiences without leaving their current pathway. Whether its for a login form, image gallery, or a complex decision tree, modals are crucial for enhancing user engagement without disrupting the flow of the application. This guide will unveil the secrets to crafting reusable and efficient modals using React, turning them into dark yet delightful components of your UI arsenal.
Why Use Modals?
Modals are not just UI elements; they are strategic tools used to capture attention, deliver information, and gather input from users at critical moments without losing the overall context of the page. Their advantages include:
- Focus and Retention: Modals focus user attention on a single task or message, which is essential for guiding users through login processes, forms, or important notices.
- Seamless Integration: They integrate seamlessly into applications, enhancing the user experience by providing interactions without loading new pages.
- Reusable Components: Designed correctly, modals can be reusable components, adaptable to various content types and interaction models within your application.
React Modal Basics
To start with, React doesnt provide built-in modal functionality, but its component architecture makes it ideal for creating highly reusable modal components. Heres a simple example of how you can create a basic modal component in React:
import React, { useState } from 'react';const Modal = ({ isOpen, children, onClose }) => { if (!isOpen) return null; return ( <div className="modal-backdrop"> <div className="modal-content"> {children} <button onClick={onClose}>Close</button> </div> </div> );};function App() { const [isModalOpen, setModalOpen] = useState(false); return ( <div> <button onClick={() => setModalOpen(true)}>Open Modal</button> <Modal isOpen={isModalOpen} onClose={() => setModalOpen(false)}> <p>This is a dark modal!</p> </Modal> </div> );}
Enhancing Modals with Animations and Transitions
To further enchant your modals and make them a seamless part of your applications user experience, consider adding animations. You can use CSS transitions or libraries like Framer Motion to animate the modal's appearance and disappearance, providing a smoother visual transition:
.modal-backdrop { position: fixed; top: 0; left: 0; right: 0; bottom: 0; background-color: rgba(0,0,0,0.5); display: flex; justify-content: center; align-items: center; transition: opacity 0.3s ease;}.modal-content { background: white; padding: 20px; border-radius: 8px; transition: transform 0.3s ease;}
Advanced Modal Scenarios
As your applications grow more complex, so too might your modal usage. Consider the following enhancements to make your modals more robust and adaptable:
- Accessibility: Ensure that your modals are accessible by implementing focus management and keyboard interactions (e.g., closing the modal on
Esc
key press). - Dynamic Content: Extend the modal component to handle dynamic content and actions, allowing for a variety of uses from a single modal structure.
- Global State Management: For applications with multiple and varied modals, manage modal states globally using React context or state management libraries like Redux or MobX.
Conclusion: Mastery of the Dark Arts
Crafting reusable modals in React is an art that combines functionality, user experience, and code reusability. By building modals that are not only functional but also adaptive and maintainable, you enhance the user experience across your entire application. Modals are powerful tools in your UI toolkit, and mastering their implementation can significantly impact the effectiveness of your application.
Experiment with different modal designs and functionalities to discover what works best for your React applications. Share your most creative modal implementations in the comments, and if you found this guide helpful, consider sharing it with your fellow developers. Dive deeper into the dark modal arts and see what magic you can conjure up in your projects!
Original Link: https://dev.to/kigazon/the-dark-modal-crafting-reusable-react-modals-1h8i

Dev To
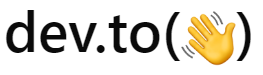
More About this Source Visit Dev To