An Interest In:
Web News this Week
- April 27, 2024
- April 26, 2024
- April 25, 2024
- April 24, 2024
- April 23, 2024
- April 22, 2024
- April 21, 2024
Assigning Functions in JavaScript: Direct vs. Through Variables
Assigning Functions in JavaScript: Direct vs. Through Variables
https://forn.fun/sh20raj/assigning-functions-in-javascript-direct-vs-through-variables-4jib
https://sh20raj.com/sh20raj/assigning-functions-in-javascript-direct-vs-through-variables-4jib
In JavaScript, functions can be assigned directly or through variables, and this distinction can have several implications depending on the context. Whether it's a normal function or an async function, how you assign it affects its behavior and usage. Let's explore the differences and possibilities.
1. Direct Assignment:
When a function is defined without assigning it to a variable, it's typically used for immediate execution or as a callback. Here's how it looks:
// Directly assigned normal functionfunction myFunction() { // Function body}// Directly assigned async functionasync function myAsyncFunction() { // Async function body}
Key Points:
Immediate Execution: These functions are often immediately executed or used as callbacks in event listeners, timeouts, or promise resolutions.
Scoped to the Parent: Directly assigned functions are scoped to the parent context. They cannot be reused elsewhere in the code.
2. Assignment through Variables:
Assigning functions to variables allows for reuse and flexibility in their usage throughout the codebase. Here's how it looks:
// Assigning a normal function to a variablelet myFunction = function() { // Function body};// Assigning an async function to a variablelet myAsyncFunction = async function() { // Async function body};
Key Points:
Reusability: Functions assigned to variables can be reused multiple times in the codebase, enhancing maintainability and reducing redundancy.
Passing as Arguments: They can be passed as arguments to other functions or stored in data structures like arrays or objects.
Async Function Consideration: When assigning async functions to variables, remember that you're storing a reference to a function that returns a promise. This allows you to use
await
within the function, but you'll need to handle the asynchronous nature appropriately.
Examples:
Let's illustrate with some examples:
Direct Assignment:
// Immediate execution of a normal function(function() { console.log("Directly assigned function executed immediately.");})();// Directly assigned function as a callbackdocument.addEventListener("click", function() { console.log("Click event handled by directly assigned function.");});
Assignment through Variables:
// Reusable function assigned to a variablelet greet = function() { console.log("Hello, world!");};// Calling the function multiple timesgreet();greet();// Passing the function as an argumentfunction callFunction(func) { func();}callFunction(greet);
Async Function Assignment:
// Async function assigned to a variablelet fetchData = async function() { let response = await fetch("https://api.example.com/data"); let data = await response.json(); console.log(data);};// Calling the async functionfetchData();
Conclusion:
In summary, the choice between directly assigning functions and assigning them to variables depends on the specific requirements of your code. Direct assignment is suitable for immediate execution or one-time use, while assigning functions to variables offers reusability and flexibility. Whether it's a normal function or an async function, understanding these distinctions will help you write more efficient and maintainable JavaScript code.
By comprehensively covering these points, you'll be well-prepared to address any questions about the differences and implications of assigning functions directly versus through variables in JavaScript interviews or discussions.
Original Link: https://dev.to/sh20raj/assigning-functions-in-javascript-direct-vs-through-variables-4jib

Dev To
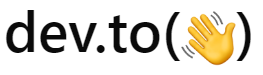
More About this Source Visit Dev To