An Interest In:
Web News this Week
- April 28, 2024
- April 27, 2024
- April 26, 2024
- April 25, 2024
- April 24, 2024
- April 23, 2024
- April 22, 2024
How to Integrate PayPal with HTML, CSS and Javascript
Have you ever visited a website and then wondered how transactions take place online, either in purchasing goods, or services, paying for an online course, or even donating online?
I got the answer to your question,
In this article, we are going to create a donation website such that a user can input their donation amount and donate through Paypal.
What is Paypal?
PayPal is an online payment system that allows individuals and businesses to send and receive payments electronically. It was founded in 1998 and has since become one of the most widely used payment platforms in the world.
PayPal enables users to create accounts and link them to their bank accounts, credit cards, or debit cards. Users can then use their PayPal accounts to make purchases or receive payments for goods and services, transfer funds to other users, or withdraw funds to their linked bank accounts.
PayPal is popular for its ease of use, security features, and wide acceptance across many online merchants and services. It is available in over 200 markets and supports more than 100 currencies, making it a convenient payment option for people and businesses worldwide.
How to Integrate PayPal into Your Product Pages
Now that we know what we want to achieve, lets get into action. I have broken down the process into 6 easy-to-follow steps. They are:
- Access PayPals developer tools to get your APIs.
- Set up a PayPal sandbox environment.
- Create and customize your PayPal button.
- Integrate your button into your current files.
- Test the integration using the PayPal sandbox environment.
- Go live and start accepting real payments.
Accessing PayPals Developer Tools to Get Your APIs
If you already have one, just hop straight to the PayPal developer tools.
To create a PayPal account, you'll need to visit the PayPal website and follow the on-screen instructions to sign up. You'll be asked to provide some personal and financial information, such as your name, email address, and credit card details.
Once you've completed the sign-up process, you'll be able to log in to your PayPal account and access the developer tools and APIs needed for integration. It's important to use a valid email address as you will receive important information and updates from PayPal.
Then, you can find the developer tools at the top right of your PayPal dashboard.
Setting Up a PayPal Sandbox Environment
The Sandbox environment is a testing environment that mimics the live PayPal environment. It allows you to test your integration before going live, which is a great feature from PayPal to ensure that everything is working as expected.
To set up a PayPal sandbox environment, you'll need to log in to your PayPal account and navigate to the developer dashboard.
From there, you can create a new sandbox account by following the on-screen instructions.
Once the sandbox account is created, you'll be able to use it to test your integration by making test payments. You can use the sandbox account in parallel with your live account.
Creating Your PayPal Button
This step involves creating a Paypal button using the PayPal SDK
<script src="https://www.paypal.com/sdk/js?client-id=YOUR_CLIENT_ID" ></script>
Add the Paypal button that will be included in the HTML file.
Add an input field that will take the amount.
<!DOCTYPE html><html lang="en"><head> <script src="./script.js" defer></script> <title> Paypal Integration with Javascript </title> <script src="https://www.paypal.com/sdk/js?client-id=YOUR_CLIENT_ID" ></script></head><body> <h1>Hello world, This is PayPal Integration with Javascript</h1> <input id="donate-amount" type="Enter Amount"> <div id="paypal-button-container"> </div></body></html>
Creating Javascript Code to invoke the Paypal buttons
Create a script.js
file and add the following function.
function initPayPalButton() { paypal .Buttons({ style: { shape: "rect", color: "gold", layout: "vertical", label: "paypal", }, createOrder: function (data, actions) { const userInput = document.getElementById("donate-amount").value; const paypalAmount = parseFloat(userInput) ; return actions.order.create({ purchase_units: [ { amount: { currency_code: "USD", value: paypalAmount } }, ], }); },}initPayPalButton();
The above function creates Paypal instance and create an order instance that takes the input value and maps it to the value to be deducted in the fundraise website
Adding an approve message
When the Paypal transaction evaluates to true, send a message to a user.
onApprove: function (data, actions) { return actions.order.capture().then(function (orderData) { // Full available details console.log( "Capture result", orderData, JSON.stringify(orderData, null, 2) ); // Show a success message within this page, for example: const element = document.getElementById("paypal-button-container"); element.innerHTML = ""; element.innerHTML = "<h3>Thank you for your payment!</h3>"; // Or go to another URL: actions.redirect('thank_you.html'); }); }, onError: function (err) { console.log(err); },
The below code is the whole Javascript implementation guide.
function initPayPalButton() { paypal .Buttons({ style: { shape: "rect", color: "gold", layout: "vertical", label: "paypal", }, createOrder: function (data, actions) { const userInput = document.getElementById("donate-amount").value; const paypalAmount = parseFloat(userInput) / 100; return actions.order.create({ purchase_units: [ { amount: { currency_code: "USD", value: paypalAmount } }, ], }); }, onApprove: function (data, actions) { return actions.order.capture().then(function (orderData) { // Full available details console.log( "Capture result", orderData, JSON.stringify(orderData, null, 2) ); // Show a success message within this page, for example: const element = document.getElementById("paypal-button-container"); element.innerHTML = ""; element.innerHTML = "<h3>Thank you for your payment!</h3>"; // Or go to another URL: actions.redirect('thank_you.html'); }); }, onError: function (err) { console.log(err); }, }) .render("#paypal-button-container");}initPayPalButton();
Hope this article helps, Leave a comment or a like,
This is the source code https://github.com/Evans-mutuku/PayPal-Intergration-Javascript-.git
Article originally published at https://melbite.com/melbite/Integrating-Paypal-with-javascript
Happy coding!
Original Link: https://dev.to/evansifyke/how-to-integrate-paypal-with-html-css-and-javascript-2mnb

Dev To
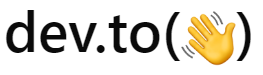
More About this Source Visit Dev To