An Interest In:
Web News this Week
- April 2, 2024
- April 1, 2024
- March 31, 2024
- March 30, 2024
- March 29, 2024
- March 28, 2024
- March 27, 2024
React Interview Questions asked to me with answers.
Question: Can you provide an overview of your experience with React? Answer: I have experience building web applications using React, including creating reusable components and managing application state with hooks and the Context API. I have also worked with popular libraries such as Redux and React Router.
Question: Can you explain the purpose of the ref attribute in the lifecycle of a functional component in React? Answer: The ref
attribute in React is used to store a reference to a DOM element or a class-based component instance. It can be used to access the properties of the element, such as its value or checked state, or to invoke methods on the element. In the lifecycle of a functional component, the ref
attribute can be used to access the DOM element after it has been rendered.
Question: Can you explain the purpose and use of the Context API in React? Answer: The Context API in React is a way to pass data through the component tree without having to pass props down manually at every level. It allows components to access data that is out of reach of their parent-child hierarchy. The Context API consists of a Provider component, which provides the data, and a Consumer component, which consumes the data.
Question: How does Redux differ from the useState hook in React, and when should we use one over the other? Answer: Redux is a state management library that allows for the centralization of application state and the management of complex application state changes. It uses a unidirectional data flow and provides a single source of truth for application state. The useState
hook, on the other hand, is a way to add state to functional components in React. It is a simpler alternative to Redux and is suitable for managing small or simple pieces of state. We should use Redux when we need to manage complex or global application state, and use the useState
hook for simpler state management needs.
Question: Can you describe the different types of components in React? Answer: In React, there are two main types of components: function-based components and class-based components. Function-based components are simple JavaScript functions that accept props as an argument and return a JSX element. Class-based components are JavaScript classes that extend the React.Component
class and include a render
method that returns a JSX element. There are also pure components, which are class-based components that optimize performance by shallowly comparing props and state before rendering, and higher-order components, which are functions that take a component as an argument and return a new component.
Question: Can you explain the concept of a virtual DOM in React and its benefits? Answer: The virtual DOM (VDOM) in React is a lightweight in-memory representation of the actual DOM. When a components state or props change, React compares the VDOM with the previous VDOM and only updates the parts of the DOM that have changed, rather than updating the entire DOM tree. This optimization helps to improve the performance of React applications by reducing the number of DOM manipulations required.
Question: Is the shadow DOM the same as the virtual DOM? Answer: No, the shadow DOM is a separate DOM tree that is attached to an element and is not accessible to the main DOM tree. It is used to encapsulate the style and behavior of a web component, making it easier to maintain and reuse. The virtual DOM, on the other hand, is an in-memory representation of the actual DOM that is used by React to optimize DOM manipulation and improve performance.
Question: Can you list some common lifecycle methods in React? Answer: Some common lifecycle methods in React are:
componentDidMount
: This method is called after a component has been rendered to the DOM. It is often used to trigger network requests or set up subscriptions.shouldComponentUpdate
: This method is called before a component re-renders and can be used to optimize performance by returning false if the component does not need to update.componentWillUnmount
: This method is called before a component is unmounted and destroyed. It is often used to clean up subscriptions or network requests.
Question: Can you explain the concept of a pure component in React? Answer: A pure component in React is a class-based component that optimizes performance by shallowly comparing props and state before rendering. If the props and state of a pure component have not changed, the component will not re-render. This optimization can help to improve the performance of a React application by reducing the number of unnecessary re-renders.
Question: Can you describe the constructor in a React component and its purpose? Answer: The constructor in a React component is a special method that is called when a new instance of the component is created. It is often used to initialize state and bind event handlers. The constructor is defined by a class-based component and is called before the component is mounted.
Question: Can you explain the concept of data dependency injection in React? Answer: Data dependency injection in React refers to the practice of providing a component with the data it needs via its props, rather than the component fetching or generating the data itself. This approach can help to make components more reusable and easier to test by decoupling them from their data dependencies.
Question: Can you differentiate between function-based and class-based components in React? Answer: Function-based components in React are simple functions that accept props as an argument and return a React element. They are often referred to as stateless because they do not have their own state and rely on props for data. Class-based components, on the other hand, are defined using a class and can have their own state and lifecycle methods.
Question: Can you explain the differences between the useMemo and useRef hooks in React? Answer: The useMemo
hook is used to memoize a value that is expensive to compute so that it is only recomputed if one of its dependencies has changed. The useRef
hook is used to create a mutable ref object whose value is persisted across re-renders. It is often used to store references to DOM elements or values that need to be accessed outside of the component's render method.
Question: Can you list five popular libraries for React? Answer: Some popular libraries for React include:
Redux: A state management library for React that helps to manage the global state of a React application.
React Router: A library for declaratively managing client-side routing in a React application.
React Bootstrap: A library for using Bootstrap components in a React application.
Axios: A library for making HTTP requests in a React application.
Moment.js: A library for manipulating dates and times in a React application.
Question: What is the useState method in React? Answer: The useState
hook is a function provided by React that allows a functional component to have state. It returns an array with two elements: the current state value and a function that can be used to update the state. The useState
hook is a way to use state in functional components, which do not have a state
property like class-based components do. To use the useState
hook, you must first import it from React and then call it inside your functional component. The hook takes an initial state value as an argument and returns an array with the current state value and a function to update the state. For example:
import { useState } from 'react';function Example() { const [count, setCount] = useState(0); return ( <div> <p>The count is {count}</p> <button onClick={() => setCount(count + 1)}>Increment</button> </div> );}
In this example, the useState
hook is called with an initial state value of 0
. The hook returns an array with the current state value (count
) and a function to update the state (setCount
). The component displays the current count and includes a button that, when clicked, increments the count by calling the setCount
function with the updated count value.
one question they ask a lot.
Question: Can you provide a detailed comparison of class-based and functional components in React, including the advantages and disadvantages of each approach?
Answer: In React, a component is a piece of code that represents a part of a user interface. There are two main types of components in React: class-based components and functional components.
Class-based components are defined as JavaScript classes that extend the React.Component
base class. They have a state object, which is an object that holds data that can change and that a component can use to render dynamic elements. Class-based components also have a lifecycle, which is a series of methods that are called at different stages of the component's existence, such as when the component is first rendered or when the component is about to be destroyed. Here is an example of a class-based component:
class ExampleComponent extends React.Component { state = { count: 0 }; incrementCount = () => { this.setState({ count: this.state.count + 1 }); } render() { return ( <div> <p>The count is {this.state.count}</p> <button onClick={this.incrementCount}>Increment</button> </div> ); }}
Functional components, on the other hand, are simple JavaScript functions that take in props (short for properties) and return JSX elements. They do not have a state object or a lifecycle, so they are generally easier to write and understand than class-based components. However, they are less powerful because they cannot have state or access the components lifecycle methods. Here is an example of a functional component:
function ExampleComponent(props) { return ( <div> <p>The count is {props.count}</p> <button onClick={props.incrementCount}>Increment</button> </div> );}
Advantages of class-based components:
They can have state and lifecycle methods, which allows them to handle more complex logic and interactions.
They can be extended using inheritance, which allows you to reuse code and share behaviour between components.
Disadvantages of class-based components:
They can be more difficult to understand and write, especially for beginners, because they use a different syntax and pattern than functional components.
They can be slower to render because they have more overhead due to their class-based structure.
Advantages of functional components:
They are simpler and easier to understand than class-based components, especially for beginners.
They are generally faster to render because they have less overhead.
Disadvantages of functional components:
They do not have state or lifecycle methods, which means they are less powerful and flexible than class-based components.
They cannot be extended using inheritance, which can make it more difficult to reuse code and share behaviour between components.
In general, it is a good idea to use functional components whenever possible because they are simpler and faster. However, if you need to add state or lifecycle methods to a component, you will need to use a class-based component.
This article is published w/ Scattr
Original Link: https://dev.to/viveksinra/react-interview-questions-asked-to-me-with-answers-1d04

Dev To
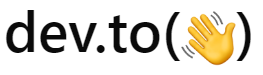
More About this Source Visit Dev To