An Interest In:
Web News this Week
- April 2, 2024
- April 1, 2024
- March 31, 2024
- March 30, 2024
- March 29, 2024
- March 28, 2024
- March 27, 2024
January 9, 2023 01:18 am GMT
Original Link: https://dev.to/elhamnajeebullah/react-typescript-how-to-use-context-api-and-usereducer-with-firestore-database-1oik
React & TypeScript: How to use Context API and useReducer with Firestore Database?
Here is an example of how you could create a React Context API and use the useReducer hook with TypeScript to manage state for a list of Amazon products stored in a Firestore database and more explanation for the code.
import React, { createContext, useReducer, Reducer, useEffect } from 'react';import { db } from './firebase';// Create a context for the productsexport const ProductsContext = createContext<{ products: Product[]; dispatch: React.Dispatch<ProductAction>;}>({ products: [], dispatch: () => {},});// Define the types for the state and actionstype Product = { id: string; name: string; price: number;};type ProductAction = | { type: 'ADD'; product: Product } | { type: 'DELETE'; id: string } | { type: 'UPDATE'; id: string; product: Product } | { type: 'SET'; products: Product[] };// Define the reducer functionconst productsReducer: Reducer<Product[], ProductAction> = (state, action) => { switch (action.type) { case 'ADD': return [...state, action.product]; case 'DELETE': return state.filter((product) => product.id !== action.id); case 'UPDATE': return state.map((product) => product.id === action.id ? action.product : product ); case 'SET': return action.products; default: return state; }};// Create a provider component for the products contextconst ProductsProvider: React.FC = ({ children }) => { const [products, dispatch] = useReducer(productsReducer, []); // Load the products from the Firestore database when the component mounts useEffect(() => { const unsubscribe = db.collection('products').onSnapshot((snapshot) => { const products: Product[] = []; snapshot.forEach((doc) => { products.push({ id: doc.id, ...doc.data() }); }); dispatch({ type: 'SET', products }); }); // Unsubscribe from the snapshot listener when the component unmounts return () => unsubscribe(); }, []); // Return the products context provider with the current state and dispatch function return ( <ProductsContext.Provider value={{ products, dispatch }}> {children} ...
This is just to show you how to use React and Typescript with Context API and useReducer hook,not a complete app.
Got any questions, please leave a comment.
Original Link: https://dev.to/elhamnajeebullah/react-typescript-how-to-use-context-api-and-usereducer-with-firestore-database-1oik
Share this article:
Tweet
View Full Article

Dev To
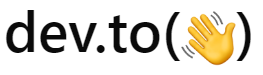
More About this Source Visit Dev To