An Interest In:
Web News this Week
- April 3, 2024
- April 2, 2024
- April 1, 2024
- March 31, 2024
- March 30, 2024
- March 29, 2024
- March 28, 2024
How to Get Started With React A Beginner's Guide Part -2
If you have read my first blog then you have got some tips about starting with react js. Today let's learn more about react js and start creating your web apps.
Why react js ?
-> React js is very popular library and it is popular because you can create your app with minimal code meaning you can write code once and reuse it again and again so the development would be faster and also react uses some extensions like jsx which lets you use html tags with javascript so that you have no need to append or manipulate dom , in simple terms React js is declarative.
Virtual vs Real Dom.
-> If you are familiar with Js then you might know about DOM. Dom is basically a Document model in browser that contains the html elements or tags. Basically with help of js we manipulate those DOM elements to create an awesome user experience. So in react ,the concept of virtual dom is quite interesting.React js creates a virtual dom that takes all our work rather than in real dom and when we work during development our components are rendered as tree structure in virtual dom.React then compares out virtual dom with real-dom and only changes those components that's need to be changed meaning it doesn't change all the code overtime so , our app would render much faster than the ordinary , typical websites. for eg , if you create a component and render the date in the component then you can find the component only is ticking rather than the whole page.
To get start with react there is a package so simply create
your development server with below code
npx create-react-app my-app
Now , you have created a react app named as my-app
now if you go to the package.json -> scripts you can find something like this,
"scripts": { "start": "react-scripts start", "build": "react-scripts build", "test": "react-scripts test", "eject": "react-scripts eject" },
- Start -> It starts your react app eg, npm start
- Build -> It build your app for production and genrates a static file. eg, npm run build,
- Test -> It is used to test you app or any components.
- Eject -> If you want to revert the app then eject.
so, when we do npm start , the development start's in port 3000;
Now you can find the main file index.js that renders our whole app using react-dom. The code looks like this.
import React from 'react';import ReactDOM from 'react-dom/client';import './index.css';import App from './App';import reportWebVitals from './reportWebVitals';const root = ReactDOM.createRoot(document.getElementById('root'));root.render( <React.StrictMode> <App /> </React.StrictMode>);
Here, basically we have appended our app component to the div tag.There is only one html tag with
.We take this root and append our js inside this div tag.
Now , our App.js we will be rendered and it is termed as a component in react js.
1. Component in react js.
-> In react js ,component is termed as the building block. We create small components and later enscapulate them to create a complex UI.Component basically takes props as input and returns jsx.
In App.js,
const App = (some props) => { return ( // it returns jsx // <> </> this is and react fragment. <>This an simple app</> )}
Here , we created an simple App component and hence some rules of component are :
- Use first letter of component as capital eg : App , Navbar etc. else it will be treated as regular functions.
- Call the component by close the tag else JSX treat it as error.
- You can nest as much as components as you want.
for eg :
export const Navbar = () => {return (<div>This is a navbar</div>)};export const App = () => {return (<App />)}
2. JSX
-> JSX is simply an javascript xml that helps to use html and javascript in single place
for eg :
const App = () => { const name = "rajiv"; return ( <h1>My name is {name}</h1>)}
Some rules while using JSX are :
a. Component should have only one div tag.
eg:
const App = () => {return ( <>Hello</> <>Wow</>)};
It throws error so , it would be wrap on single tag.
b. Jsx is compiled to normal html by babel.
Conclusion,
I guess now you can get started and I will bring part-3. Till then if any problem consult me ,
https://www.facebook.com/Rajiv.chaulgain/,
https://github.com/rajivchaulagain
Original Link: https://dev.to/rajivchaulagain/how-to-get-started-with-react-a-beginners-guidepart-2-269m

Dev To
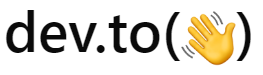
More About this Source Visit Dev To