An Interest In:
Web News this Week
- April 27, 2024
- April 26, 2024
- April 25, 2024
- April 24, 2024
- April 23, 2024
- April 22, 2024
- April 21, 2024
Exploring Feature Flag use AWS AppConfig
Hello Everyone!
This week, I learned about AWS AppConfig, especially for Feature Flag. I need feature flags for my applications since I use Trunk Based Development. Let's move on.
Setup AWS AppConfig
You may setup your AWS AppConfig use this official guide. You need to follow the 6 steps from the guide:
- Create an AWS AppConfig application
- Create an environment
- Create configuration profiles and feature flags
- Create a deployment strategy
- Deploying a configuration
- Retrieving the configuration
In this article, I will focus on step 6 and the code.
Retrieve the Feature Flag from AWS AppConfig
Before you retrieve the Feature Flag, you will need AWS_ACCESS_KEY_ID
and AWS_SECRET_ACCESS_KEY
with appropriate access. I have created a "small library" to call the feature flag and use AWS SDK for Javascript.
import { AppConfigDataClient, BadRequestException, GetLatestConfigurationCommand, StartConfigurationSessionCommand,} from "@aws-sdk/client-appconfigdata";const client = new AppConfigDataClient({});let existingToken: string;const getToken = async (): Promise<string> => { const getSession = new StartConfigurationSessionCommand({ ApplicationIdentifier: process.env.APP_CONFIG_APP_IDENTIFIER, ConfigurationProfileIdentifier: process.env.APP_CONFIG_CONFIG_PROFILE_IDENTIFIER, EnvironmentIdentifier: process.env.APP_CONFIG_ENVIRONMENT_IDENTIFIER, }); const sessionToken = await client.send(getSession); return sessionToken.InitialConfigurationToken || "";};const featureFlag = async (flag: string): Promise<boolean> => { if (!existingToken) { existingToken = await getToken(); console.log(existingToken); } try { const command = new GetLatestConfigurationCommand({ ConfigurationToken: existingToken, }); const response = await client.send(command); let flags: any = {}; if (response.Configuration) { let str = ""; for (let i = 0; i < response.Configuration.length; i++) { str += String.fromCharCode(response.Configuration[i]); } const allFlag = JSON.parse(str); console.log(allFlag); flags = Object.assign({}, allFlag); } return Boolean(flags[flag]?.enabled); } catch (err) { if (err instanceof BadRequestException) { existingToken = await getToken(); console.log(existingToken); // recall return featureFlag(flag); } else { throw err; } }};export default featureFlag;
Code Explanation: For getting my feature flag, I need to call GetLatestConfiguration
API. Before I call that API, I need to get my configuration session token (use getToken
). If you want to check my code. I've published the code in Github and the library to .
/
Explore Feature Flag using AWS AppConfig
feature-flag
Explore Feature Flag using AWS AppConfig
Environment Variables
AWS_REGION="ap-southeast-1"AWS_ACCESS_KEY_ID=""AWS_SECRET_ACCESS_KEY=""APP_CONFIG_APP_IDENTIFIER=""APP_CONFIG_CONFIG_PROFILE_IDENTIFIER=""APP_CONFIG_ENVIRONMENT_IDENTIFIER=""
How to try?
- Setup Environment
- Modify
demo/index.ts
with your flag. - Run
npm install
oryarn
- Run
yarn dev
ornpm run dev
LICENSE
MIT
MIT LicenseCopyright (c) 2022 Bervianto Leo PratamaPermission is hereby granted, free of charge, to any person obtaining a copyof this software and associated documentation files (the "Software"), to dealin the Software without restriction, including without limitation the rightsto use, copy, modify, merge, publish, distribute, sublicense, and/or sellcopies of the Software, and to permit persons to whom the Software isfurnished to do so, subject to the following conditions:The above copyright notice and this permission notice shall be included in allcopies or substantial portions of the Software.THE SOFTWARE IS PROVIDED
Note: Please don't use this for production. This library is still in heavy development and experimental. I want to improve this library before becoming production-ready.
How to call my library?
- Promise then/catch
import featureFlag from '@berviantoleo/feature-flag';featureFlag('try_feature_flag').then((result) => { console.log(result);});
You may use async
/await
approach too.
- Example output
Welcome to contribute to my small library/project. :) If you have any inputs, feel free to add them here too.
What's Next?
I'm going to create a similar library/project for .NET. So stay tuned!
Thank you
Source from unsplashOriginal Link: https://dev.to/aws-builders/exploring-feature-flag-use-aws-appconfig-9f9

Dev To
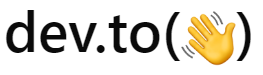
More About this Source Visit Dev To