An Interest In:
Web News this Week
- April 27, 2024
- April 26, 2024
- April 25, 2024
- April 24, 2024
- April 23, 2024
- April 22, 2024
- April 21, 2024
April 14, 2022 04:10 am GMT
Original Link: https://dev.to/bipon68/user-create-using-nodejs-16hb
User create using NodeJS
Objective: In this article, you will know mongoose model, how to create user using node, router.
Pre-requisite Prior to completing this article, you should have already installed all pre-requisite tooling including: Visual Studio Code, Node Package Manager (NPM), Node, Postman, Mongo Compass.
Create a Model (ProfileModel.js)
const mongoose = require('mongoose')const DataSchema = mongoose.Schema({ FirstName : {type: String}, LastName : {type: String}, EmailAddress : {type: String}, MobileNumber : {type: String}, City : {type: String}, UserName : {type: String}, Password : {type: String}});const ProfileModel = mongoose.model('Profile', DataSchema)module.exports = ProfileModel;
Create a Controller (ProfileController.js)
At first import ProfileModel. Declare a variable reqBody to store body data. Then create user using ProfileModel model
const ProfileModel = require("../models/ProfileModel");exports.CreateProfile = (req, res) => { let reqBody = req.body; ProfileModel.create(reqBody, (err, data) => { if(err){ res.status(400).json({status: "Failed to user create", data: err}) }else{ res.status(200).json({status: "Successfully user created", data: data}) } })}
Default configuration (app.js)
// Basic importconst express = require('express');const router = require('./src/routes/api')const app = new express();const bodyParser = require('body-parser')// Database lib importconst mongoose = require('mongoose')// Body parser implementapp.use(bodyParser.json())// MongoDB database connectionlet uri = 'mongodb://127.0.0.1:27017/PracticeDB'let options = {user: '', pass: ''}mongoose.connect(uri, options, (err) => { if(err){ console.log(err) }else{ console.log('Database Connection Success') }})// Routing Implementapp.use('/api/v1', router)// Undefined Route Implementapp.use("*", (req, res) => { res.status(404).json({status: "Failed", data: "Not Found"})})module.exports = app;
Routes configuration (api.js)
const express = require('express');const ProfileController = require('../controller/ProfileController')const router = express.Router();router.post('/CreateProfile', ProfileController.CreateProfile)module.exports = router;
Index file (index.js)
const app = require('./app')app.listen(5000, function(){ console.log('Server run at @5000 port')})
Now open the Postman and configure few thing like
Then create a user giving basic information and click Send Button
Thanks for reading. Happy journey.
Original Link: https://dev.to/bipon68/user-create-using-nodejs-16hb
Share this article:
Tweet
View Full Article

Dev To
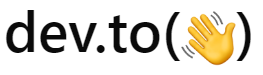
More About this Source Visit Dev To