An Interest In:
Web News this Week
- April 27, 2024
- April 26, 2024
- April 25, 2024
- April 24, 2024
- April 23, 2024
- April 22, 2024
- April 21, 2024
Different ways of storing data using NodeJS and KnexJS
I created lots of functions with different structures but with similar idea. One of them is about storing data into the database.
Approach 1:
async store ({ firstName, lastName }) { try { const [id] = await knex('users') .insert({ first_name: firstName, last_name: lastName }) return id } catch (error) { throw error }}
Usage:
await store({ firstName: 'Ernie Jeash', lastName: 'Villahermosa'})
This approach however gets messy when the column count increases.
Approach 2:
async store (payload) { try { const [id] = await knex('users') .insert(payload) return id } catch (error) { throw error }}
Usage:
await store({ first_name: 'Ernie Jeash', last_name: 'Villahermosa'})
The 2nd approach does not create messy and accepts both array and object but is vulnerable and easily creates error if you have an unrecognized column.
Approach 3
const _pickBy = require('lodash/pickBy')async store (payload) { try { const fillables = ['first_name', 'last_name'] const [id] = await knex('users') .insert(_pickBy(payload, col => fillables.includes(col))) return id } catch (error) { throw error }}
Usage:
await store({ first_name: 'Ernie Jeash', last_name: 'Villahermosa'})
This approach is similar to the second approach but is less error prone because the unregistered properties are omitted. But it only accepts an object. Not versatile enough.
Final Approach
const _castArray = require('lodash/castArray')const _pickBy = require('lodash/pickBy')const _isNil = require('lodash/isNil')async store (payload) { const fillables = new Set([ 'user_id', 'admin_id' ]) try { const data = _castArray(payload) .map(hay => _pickBy(hay, (val, key) => { return !_isNil(val) && fillables.has(key) })) const [id] = await store.knex('users').insert(data) return id } catch (error) { throw error }}
Usage:
await store({ first_name: 'Ernie Jeash', last_name: 'Villahermosa'})// orawait store([ { first_name: 'Ernie Jeash', last_name: 'Villahermosa' }, { first_name: 'Marielle Mae', last_name: 'Valdez' }])
This approach takes advantage of ES6's Set
which is faster at lookups. It accepts both array and object. At the same time, it omits unregistered properties which solved the previous approach's problems. I also used a few functions from lodash.
PS: I'm open to new suggestions
Original Link: https://dev.to/karmablackshaw/different-ways-of-storing-data-using-nodejs-and-knexjs-4723

Dev To
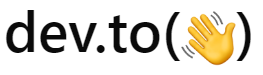
More About this Source Visit Dev To