An Interest In:
Web News this Week
- April 27, 2024
- April 26, 2024
- April 25, 2024
- April 24, 2024
- April 23, 2024
- April 22, 2024
- April 21, 2024
Common Git Mistake Fixes
Everybody makes mistakes and everybody has those days especially when it comes to git, so here is a summary of solutions to common git errors (although of course there may be many approaches).
Circumstance: Made code changes and/or staged changes before creating a new branch
- we either made changes to our code or made changes and staged those changes to our index while in a wrong branch
If we made changes to our code before branching off of our starter branch (for ex: main/master) or we made changes and staged those changes, we can run git status
to check which files contain changes.
Since we havent committed our changes to our current wrong branchs history yet, we can simply run git checkout -b new_branch_name
and our changes (staged or not) will follow us to whichever new branch we just created. From our new branch, we can then add and commit our changes to this new branchs git history.
Weve tackled a slip up for when weve been wanting to create a new branch; but, if we want to move our changes to a pre-existing branch, we would need to follow some kind of stashing workflow.
Circumstance: Made code changes and/or staged changes before checking out a pre-existing branch
- we made changes to our code and forgot to checkout a pre-existing branch
- we may have added/staged our changes as well
If we made code changes in the wrong branch but those changes havent been committed yet, we can use a git stash
workflow to shelve/stash our changes before we checkout the proper pre-existing branch.
git stash
Workflow
When running git status
we can view which file changes we would like to be taking with us to the proper branch. Then, we can run git stash
, which will stash/shelve all of our current changes (whether added/staged or not). After stashing, we can checkout the pre-existing proper branch with git checkout <correct_branch>
. Once were in the proper branch, we can run git stash pop
to pop all of our changes out of the stash/shelf. After popping, we would be able to continue our usual git add
and git commit
workflow to add and commit those changes to the proper branch.
Note that if we had changes staged before our stash and we later popped our stashes, those staged changes would be un-staged on-pop automatically.
So the full workflow would look like:
git status
to check which changes we would be stashinggit stash
to stash/shelf all of our changesgit checkout <branch>
to checkout the proper branchgit stash pop
to remove the most recent stash from our shelf and track those changes in our proper branchgit add
&git commit
workflow to add/stage and commit specific files to our proper branchs history
If we altogether committed in the wrong branch, we can use a resetting workflow.
Circumstance: Committed in the wrong branch
- we made changes to the code
git add .
we staged all of our workgit commit -m "my first commit!"
we committed our workgit branch
we check our branch and OH NO! We realize we were in the wrong branch all along
If we added and committed our changes to the wrong branch locally, we could always rollback however many commits we need and move our un-staged changes into a new branch.
First, we would run git log
to check our commit history and view how many commits we would like to undo.
Then, we would run git reset HEAD~N
where N
is the number of our commits to undo.
If we do git log
again, we can see that our commits are no longer in the history of the current wrong branch. When we do git status
, we can also sanity check that the changes have been tracked and are ready to be staged.
Because we would be checking out a new branch, we can simply run git checkout -b new-correct-branch
where new-correct-branch
is the new branch we wish to track, stage, and commit our changes in. Note: if were checking out a pre-existing branch, we would probably need to run our git stash
workflow to move our changes to the proper pre-existing branch.
Once were in our new-correct-branch
, we can run git add <paths-for-files-we-wish-to-add>
(or we can also run git add .
to add all of our changes that appear when we run git status
). And then we can run our git commit -m "insert commit message here"
to finally commit our changes to the proper branch.
If we run git log
, we should see the proper commit history in our proper branch.
So overall the workflow would look like so:
git log
to check our commit history to determine the number of commits we would like to roll backgit reset HEAD~N
to reset the head of our current wrong branch back by our previously determined N number commitsgit log
to sanity check that our commit history no longer contains the wrong commitsgit status
to sanity check that our changes are being tracked and ready to move with us to whichever branch we checkoutgit checkout -b new-correct-branch
to create and move into our new proper branch that will store our accurate commit historygit add <file-paths>
to stage our desired filesgit commit -m "insert commit message here"
to commit our changes to the proper branch historygit log
to sanity check our current proper branchs commit history
Sometimes our mistakes aren't related to where our changes were made. Sometimes we pulled down the wrong branch altogether, so then what?
Circumstance: Pulled the down the wrong branch
- thinking were in branch1, we
git pull origin branch2
and OH NO! We realize we pulled down the wrong branch from the remote to our local branch
If we pull down the wrong remote branch to our local branch, we can always run a hard reset of the current branch with git reset --hard origin/current_branch
. Note of caution: reset --hard
is destructive in nature, so it will remove all staged and un-staged changes to overwrite the current local branch.
If we want to save our changes somewhere before we do a hard reset of the branch, we can use the git stash
workflow mentioned above.
TLDR:
- We shouldnt get into the habit of making mistakes, but there are ways to fix them
- We can always checkout a new branch if weve made staged or un-staged changes in a wrong branch as long as we dont commit those changes yet
- If were trying to move our changes to a pre-existing branch,
git stash
is a pretty useful command for shelving our changes temporarily - If we ever do commit our changes to the wrong branch, we could always run a reset of any branch to rollback commits (and then continue to move our staged or un-stanged changes to the proper branch)
- If we ever pull down the wrong branch, we could run a hard reset
For Funsies
Here are some extra useful commands:
git stash list
to view all of the stashes in our shelfgit stash pop <stashID>
to pop a specific stashgit stash drop <stashID>
to delete a stash from our shelf use with cautiongit reset -- <filepath>
to un-stage a specific file from our indexgit checkout <filepath>
to undo/delete all changes of a specific file use with caution
Resources:
- git stash documentation: https://git-scm.com/docs/git-stash
- git reset documentation: https://git-scm.com/docs/git-reset
- git checkout documentation: https://git-scm.com/docs/git-checkout
Original Link: https://dev.to/adelinealmanzar/common-git-mistake-fixes-56ac

Dev To
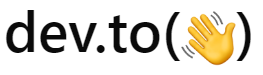
More About this Source Visit Dev To