An Interest In:
Web News this Week
- April 27, 2024
- April 26, 2024
- April 25, 2024
- April 24, 2024
- April 23, 2024
- April 22, 2024
- April 21, 2024
Try before you buy: adding a trial period to subscriptions
Sometimes, you want to provide your customers with a Try before you buy solution. With Stripe Checkout, you can add a trial period when setting up subscriptions so your customers arent charged for a certain period of time until the subscription kicks in.
If this is something youd like to implement, lets have a look at how to do it.
Setting up a subscription with Stripe Checkout
For the purpose of this post, I'm only going to focus on the code sample responsible for creating the Checkout session and handling the trial period. If you'd like more details about the entire code needed server-side, please refer to our interactive integration builder.
The sample below shows the minimum amount of code you need to implement a standard subscription functionality (in Node.js):
const session = await stripe.checkout.sessions.create({ billing_address_collection: 'auto', line_items: [ { price: '{{PRICE_ID}}', quantity: 1, }, ], mode: 'subscription', success_url: `${YOUR_DOMAIN}?success=true`, cancel_url: `${YOUR_DOMAIN}?canceled=true`,});
The Stripe documentation has code samples for a few other languages so I would recommend you check it out if youd like to use another popular programming language.
What this code does is create a Checkout session for a subscription, for a single product referenced by its price ID.
To find a product's price ID, navigate to a product's page in the Stripe dashboard, and under the Pricing section, each price should show an ID starting with price_
.
At this point, if a customer goes through the checkout process, they will be charged right away.
Adding a trial period
If you'd like to add a trial period for a subscription, you can do so by using subscription_data
to add a trial_period_days
number. This number needs to be an integer and at least equal to 1.
Overall, a subscription with a trial period of 2 weeks would be written this way:
const session = await stripe.checkout.sessions.create({ billing_address_collection: 'auto', line_items: [ { price: '{{PRICE_ID}}', quantity: 1, }, ], mode: 'subscription', subscription_data: { trial_period_days: 14 }, success_url: `${YOUR_DOMAIN}?success=true`, cancel_url: `${YOUR_DOMAIN}?canceled=true`,});
If all goes well, when testing out your checkout page, you should see it mention the 14 days trial.
After this change, if a customer goes through the checkout process for this subscription, the first time they will be charged will be 14 days later.
In the Stripe dashboard, subscriptions with a trial period are indicated with a specific badge.
Creating a subscription without Stripe Checkout
If you're handling subscriptions without using Stripe Checkout, there's an additional way to indicate a trial period.
First, the sample below shows how to create a subscription using a customer ID and price ID.
const subscription = await stripe.subscriptions.create({ customer: 'cus_111aaa222bbb', items: [ { price: 'price_333ccc444ddd', }, ],});
Adding a trial period can be done by using trial_end
with a timestamp, for example:
const subscription = await stripe.subscriptions.create({ customer: 'cus_111aaa222bbb', items: [ { price: 'price_333ccc444ddd', }, ], trial_end: 1648760166,});
Ending a trial early
If you want to end a trial early, you can update a subscription via an API call, setting trial_end
to a new value or, now
to end immediately.
stripe.subscriptions.update('sub_555eee666fff', { trial_end: 'now',});
If you'd prefer to make the update via the Stripe dashboard, visit the subscriptions page, select the subscription you'd like to update, under the Actions dropdown, select "update subscription", modify the date in the "Free trial days" date picker and save your changes by clicking on the "update subscription" button.
That's it! With a couple of lines of code, you can offer a free trial period and give people the opportunity to test out your product(s)!
Stay connected
In addition, you can stay up to date with Stripe in a few ways:
Follow us on Twitter
Join the official Discord server
Subscribe to our Youtube channel
Sign up for the Dev Digest
Original Link: https://dev.to/stripe/try-before-you-buy-adding-a-trial-period-to-subscriptions-248d

Dev To
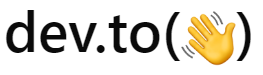
More About this Source Visit Dev To