JavaScript Made Easy: Part 7
For Part 7, we will continue talking about functions. During Part 6, we learned about inner variables (local variables) and outer variables (global variables). This is the first topic I will expand on. Open up your repl and code along!
Function scope
It is important to know that variables defined inside a function cannot be accessed from anywhere outside the function. Take this example:
// A function with local variablesfunction incrementLocalVariables() { let num1 = 2//local variable let num2 = 3//local variable num1++;//increments num1 num2++;//increments num2 return num1 + num2;//returns the variables added together}incrementLocalVariables();//calls the function
Notice that in the example above, the function has local variables. If you wanted to console.log(num1) or console.log(num2) from outside the function you could not do it. Go ahead and try it in your repl. The local variables can only be used inside of the function. You can also try putting a console.log after num1++ and num2++ to see that the variables were increased by one i.e. console.log(num1) after the variables are incremented. Now, try to copy and paste num1 and num2 outside the function and remove them from inside the function. Also, put a console.log outside the function to see the results. Like this:
let num1 = 2//a global variablelet num2 = 3//a global variable// A function with local variablesfunction incrementLocalVariables() { num1++;//increments num1 num2++;//increments num2 return num1 + num2;//returns the variables added together}incrementLocalVariables();//calls the functionconsole.log(num1);//shows the value of num1 was changed
Because programs run top to bottom and left to right this program has a certain order to it. For example, num1 and num2 were declared outside the function, then the function ran because it was called by incrementLocalVariables(). Then, the global variables were incremented by the function, then we logged the global variables after they were incremented. Go ahead and experiment with this function and moving local and global variables around. Also, try to figure out what value was returned when we ran the function.
Nested Functions
Now that we have a better understanding of scope, I would like to move on to a more advanced topic regarding scope. First, a function defined inside another function can also access all variables defined in its parent function, and any other variables to which the parent function has access. Example(inspired by the MDN Web Docs):
// The following variables are defined in the global scopelet name = 'David';// A nested function examplefunction getScore() { let num1 = 2;//local variable let num2 = 3;//local variable //inner function function add() { return name + ' scored ' + (num1 + num2); } return add();}getScore(); // Returns "David scored 5"
There is a lot going on here:
- Starting from the top, look at how the name variable is declared. This variable is in the global scope. That means it can be accessed by the inner and the outer function.
Then, we get to the getScore function which is the outer function. This function has two local variables inside of it. Those variables are num1 and num2. Both of these variables can be accessed by the inner and the outer function. However, you cannot access them and use them outside of the function. - The inner function returns a concatenated string that consists of:
the value stored in the global variable
the string ' scored' with a space in it
both local variables added together
Notice that when the local variables were added together they were put inside of parenthesis. This is similar to what you would see in arithmetic. This signifies that this operation is set apart from others. Since those variables are concatenated with the (+) operator, the result becomes part of the string.
- Finally, the return statement in the outer function consists of calling the inner function. Therefore, whatever the inner function returns becomes the return for the outer function. This is a lot to take in. Please take time to fully understand everything and experiment with it in your repl.
Further Reading
Because functions are so important, here is some additional reading on the topics we discussed in this post:
- https://www.geeksforgeeks.org/javascript-nested-functions/
- https://developer.mozilla.org/en-US/docs/Web/JavaScript/Guide/Functions
- https://www.tutorialspoint.com/javascript/javascript_nested_functions.htm
I hope you have enjoyed this post! Please check out the entire "JavaScript Made Easy" series by David Tetreau. There will be a new post daily.
Original Link: https://dev.to/dtetreau/javascript-made-easy-part-7-3gld

Dev To
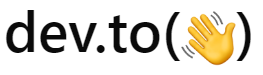
More About this Source Visit Dev To