JavaScript Made Easy: Part 5
During Part 4, we were discussing operators. We went over assignment operators and arithmetic operators. This time, we will dig a little deeper into the topic of operators.
Assignment Operators
We learned in a previous post that the assignment operator is used to assign a value to a variable by means of an equals sign. Additional assignment operators are:
+=
this operator adds to a variable and sets it equal to that new value
Example:
let gradePointAverage = 3.9;gradePointAverage += .1;console.log(gradePointAverage); // output is 4
-=
this operator subtracts from a variable and sets it to that new value
Example:
let gradePointAverage = 3.9;gradePointAverage -= .1;console.log(gradePointAverage); // output is 3.8
*=
this operator multiplies a value times a variable and sets the variable equal to that new value
Example:
let gradePointAverage = 3.9;gradePointAverage *= .1;console.log(gradePointAverage); // output is 0.39
//these work similarly to the examples above
/=
%=
**=
String Operators
If you use the plus sign or the plus equals sign to join strings together, they are considered string operators. The process by which you join them is called string concatenation. Let's see an example:
//expected output: 'I am joining two strings'console.log('I am joining ' + 'two strings');
Comparison Operators
There are several comparison operators. They compare operands to return true or false.
Equal (==)
Returns true if the operands are equal.
This operator does not check data type.
/*returns true because this operator does notcheck data type*/console.log("3" == 3);
Not equal (!=)
Returns true if the operands are not equal
/*returns false because this operator does not check data type*/console.log("3" != 3);
Strict equal (===)
Returns true if the operands are equal and of the same type.
/*returns false because the data types are different */console.log("3" === 3);
Strict not equal (!==)
Returns true if the operands are of the same type but not equal, or are of a different type.
/*returns true becausethis checks data typesand they are not the same*/console.log(3 !== "3");
Greater than (>)
Returns true if the left operand is greater than the right operand
//returns trueconsole.log(2 > 1);
Greater than or equal (>=)
Returns true if the left operand is greater than or equal to the right operand.
//returns trueconsole.log(1 >= 1);
Less than (<)
Returns true if the left operand is greater than the right operand
//returns trueconsole.log(1 < 2);
Less than or equal (<=) Returns true if the left operand is less than or equal to the right operand.
//returns trueconsole.log(1 <= 1);
Conditional (ternary operator)
Understanding this operator is as simple as saying, "If a condition is true, the operator has this value, otherwise it has that value." The actual format of this is: condition ? value1 : value2. This is just an alternate way to write the standard operators.
For example:
const speed = 70;//expected output 'not speeding'console.log((speed > 70) ? 'speeding' : 'not speeding');
There are several other types of operators. We will go over bitwise operators, type operators, and logical operators in the next post along with some new concepts!
I hope you have enjoyed this post! Please check out the entire "JavaScript Made Easy" series by David Tetreau. There will be a new post daily.
Original Link: https://dev.to/dtetreau/javascript-made-easy-part-5-5a99

Dev To
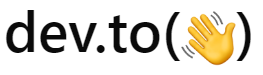
More About this Source Visit Dev To