An Interest In:
Web News this Week
- April 26, 2024
- April 25, 2024
- April 24, 2024
- April 23, 2024
- April 22, 2024
- April 21, 2024
- April 20, 2024
Creating Desktop Apps with ReactJS using Tauri
Introduction
Building desktop apps use to be difficult, first, you would need to know quite a number of languages like Java or C++ and get buried to quite a number of setups which can be wearing. Thankfully as technology progressed, frameworks like ElectronJS came into the picture and simplified the process.
In this article, Ill introduce you to Tauri, A toolchain for building native desktop apps using any HTML and JavaScript-based front-end framework, such as React, Vue.js, or Angular.
This article will be useful to you if:
- youve been building a web or mobile application and want to see how you could create a desktop app with React.Js.
- you have been building applications on the web with HTML, CSS, and JavaScript, and you want to use the same technologies to create apps targeted at Windows, macOS, or Linux platforms.
- you are already building cross-platform desktop apps with technologies like Electron, and you want to check out alternatives.
- you are a Rust enthusiast, and youd like to apply it to build native cross-platform applications.
We will look at how to build a native cross-platform application from an existing web project. Lets get to it!
Note: This article assumes youre comfortable with HTML, CSS, and React.Js.
What is Tauri.js?
The Official Tauri site describes it as;
- A framework for building tiny, blazing-fast binaries for all major desktop platforms.
- It allows a rust-sourced binary with an API that the front-end can interact with.
- Built with Rust and the CLI leverages Node.js, hence making Tauri a genuinely polyglot approach to creating and maintaining great apps. Polyglot in this context means that Tauri uses multiple programming languages such as Rust, JavaScript, and TypeScript.
- A Framework-agnostic toolchain for building highly secure native apps that have tiny binaries (i.e. file size) and that are very fast (i.e. minimal RAM usage).
Simply put, Tauri makes you utilize web technologies to create smaller and secured native desktop apps.
Electron Vs Tauri: Their differences
Electron Js has been around for a while now and as even made mass adoption amongst developers and great companies like Atom, Vscode, and more. In this section, we would compare some features that make Tauri distinct from Electron Js.
This table is from its Github page.
Features | Tauri | Electron |
---|---|---|
Binary Size MacOS | 0.6 MB | 47.7 MB |
Memory Consumption MacOS | 13 MB | 34.1 MB |
Interface Service Provider | Varies | Chromium |
Backend Binding | Rust | Node.js (ECMAScript) |
Underlying Engine | C/C++ | V8 (C/C++) |
FLOSS | Yes | No |
Multithreading | Yes | No |
Bytecode Delivery | Yes | No |
Can Render PDF | Yes | No |
Multiple Windows | Soon | Yes |
Auto Updater | Soon | Yes |
Cross-Platform | Yes | Yes |
Custom App Icon | Yes | Yes |
Windows Binary | Yes | Yes |
MacOS Binary | Yes | Yes |
Linux Binary | Yes | Yes |
iOS Binary | Soon | No |
Android Binary | Soon | No |
Localhost Server | Yes | Yes |
No localhost option | Yes | No |
Desktop Tray | Soon | Yes |
Splashscreen | Yes | Yes |
Sidecar Binaries | Yes | No |
We see from the above table that despite the fact that Tauri is pretty new it has some amazing features and even more, while others are in the pipeline.
Benefits of Tauri
The following are the reason you might want to consider using Tauri in your next Desktop app.
- Its compatible with any JavaScript framework, hence you dont have to change your stack.
- Its variety of design patterns makes it possible to get started with implementing features with easy configurations.
- Like you saw from the above table, the size of a Tauri app is just 0.60 MB (600 KB).
- Also, the memory consumption of a Tauri app is less than half of an Electron-based app. From the table, it is usually about 13 MB.
- Unlike Electron, relicensing is made possible with Tauri. Apps built with Tauri can be officially shipped into PureOS.
- The flexibility and framework agnostic ability of Tauri makes it possible to turn a web-based code-base into a native desktop app, without altering anything.
Tauri in Action!
Tauri uses Node.js under the hood to scaffold an HTML, CSS, and JavaScript rendering window as a user interface (UI), majorly bootstrapped by Rust.
The outcome is a monolithic binary that can be distributed as common file types for macOS (app/dmg), Windows (exe/MSI), and Linux (deb/app image).
How Tauri Apps Are Made
A Tauri app is created via the following steps:
- First, make an interface in your GUI framework, and prepare the HTML, CSS, and JavaScript for consumption.
- The Tauri CLI takes it and rigs the underlying native code(Rust runner) according to your configuration.
- In development mode, it creates a WebView window, with debugging and Hot Module Reloading.
- In build mode, it rigs the bundler and creates a final application(native installers) according to your settings.
Check out more of its operation on Its site.
Setting Up Your Environment
Now that you know what Tauri is and how it works, lets do the setups.
We will not be covering environment setups for any OS in this tutorials, but you can check the setup for Windows, macOS, and Linus are also available,
For those using the Windows Subsystem for Linux (WSL) please refer to its Linux specific instructions instead.
Implementing Tauri With React.JS
Now that we have Tauri installed, lets bundle an existing web project.
For this application, we would be using a Money Heist Episode Picker I built with React and Typescript. Read how I built it here.
https://codesandbox.io/s/money-heist-episode-picker-react-and-typescript-web-app-px1qe
Go ahead and fork the repository, which will serve as a shell. After forking it, make sure to clone the fork by running this:
git clone https://github.com/[yourUserName]/React-Desktop-App.git
You can install Tauri as both a local and a global dependency, but in this tutorial, well be installing it locally.
After cloning the project, run the following to install the dependencies:
#Using npmnpm install#using yarnyarn
After a successful installation of dependencies, start the server with npm start
, your app should start on http://localhost:3000
.
Defining Tauri
If you decide to use Tauri as a local package with npm, you will have to define a custom script to your package.json:
{ // Add Tauri to the script object "scripts": { "tauri": "tauri", "dev": "npm run tauri dev", "bundle": "tauri build", }
- Tauri command - defines Tauri and tells your OS, youre about to create a Tauri based app.
- dev - It enables us to start your app on our local machine.
- bundle - Running this command will build our app for production.Initialize Tauri
We will need to add an src-Tauri
directory to the root of your app directory. Inside this directory are files and folders used by Tauri to configure your desktop app.
To initialize Tauri in other for us to have this folder, run;
`npm run Tauri init`
While initializing youll be asked a few questions, answers them based on your project scope.
Heres how mine looks
After its done initializing, it will create a src-tauri
directory, lets check out the contents:
src-tauri .gitignore Cargo.toml rustfmt.toml tauri.conf.json icons 128x128.png [email protected] 32x32.png icon.icns icon.ico icon.png src build.rs cmd.rs main.rs
Cargo.toml
inside src-tauri/Cargo.toml
is like the package.json
file for Rust.
It contains configurations for our app. The content of this file is beyond the scope of this tutorial.
##Bundling our app
To bundle Money-Heist
for your current platform, simply run this:
#builds our react appnpm run build#builds the Tauri cratesnpm run bundle
Note: The first time you run this, it will take some time to collect the Rust crates and build everything, but on subsequent runs, it will only need to rebuild the Tauri crates which is much quicker.
So go grab some chocolates while its building
When the above is completed, you should have a binary of money-heist
for your current OS.
Because of the way that Cargo builds its targets, the final app is placed in the following folder:src-Tauri/target/release/money-heist
.
Yours might be src-tauri/target/release/bundle/[your-app]
.
Conclusion
In this article we have seen how cool it is to build desktop apps with React utilizing Tauri, Id like to see what you came up with the comment section.
Lemme know what you think about Tauri.
If you enjoyed the article please lets connect on twitter
Original Link: https://dev.to/krofax/creating-desktop-apps-with-reactjs-using-tauri-af

Dev To
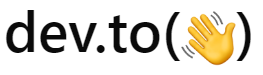
More About this Source Visit Dev To