An Interest In:
Web News this Week
- April 17, 2024
- April 16, 2024
- April 15, 2024
- April 14, 2024
- April 13, 2024
- April 12, 2024
- April 11, 2024
React.memo - Optimize React Functional Components
In React, optimizing the performance of functional components is crucial for ensuring a smooth and efficient user interface. One way to achieve this is by using the React.memo function, which is a higher-order component (HOC) that helps optimize the rendering process of these components. In this article, we'll dive into the concept of React.memo and see how it can improve the performance of your React applications.
Explanation:
React.memo is a function that allows you to memoize the result of a functional component's rendering. It works by caching the rendered output of the component and only re-rendering it if the inputs (props) to the component change. This can significantly reduce the number of unnecessary re-renders, especially when dealing with complex components or large data sets.
Example:
Let's consider a simple example where we have a functional component called "UserList" that renders a list of users. The list of users is passed as a prop to the component. Without using React.memo, any change in the parent component would result in a complete re-render of the "UserList" component, even if the user list remains the same.
import React from "react";const UserList = ({ users }) => { return ( <ul> {users.map((user) => ( <li key={user.id}>{user.name}</li> ))} </ul> );};export default UserList;
To optimize the rendering of the "UserList" component, we can wrap it with React.memo:
import React from "react";const UserList = React.memo(({ users }) => { return ( <ul> {users.map((user) => ( <li key={user.id}>{user.name}</li> ))} </ul> );});export default UserList;
Now, if the parent component re-renders but the user list remains the same, React.memo will prevent the "UserList" component from re-rendering. It will only re-render if the users prop changes.
Conclusion:
React.memo is a powerful tool for optimizing the performance of functional components in React. By memoizing the result of rendering, it reduces unnecessary re-renders and improves the overall efficiency of your application. Remember to use React.memo judiciously, especially when dealing with components that have expensive rendering processes or components that receive large data sets as props.
Follow me in X/Twitter
Original Link: https://dev.to/ashsajal/reactmemo-optimize-react-functional-components-516m

Dev To
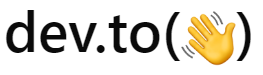
More About this Source Visit Dev To