An Interest In:
Web News this Week
- April 16, 2024
- April 15, 2024
- April 14, 2024
- April 13, 2024
- April 12, 2024
- April 11, 2024
- April 10, 2024
Some of Our Sources
View All SourcesUsing React and Firebase for Adding Data to Firestore
At itselftools.com, we have accrued substantial experience over the development of 30+ projects using technologies like Next.js and Firebase. In this article, we will dive into a specific use case demonstrating how to add data to Firestore using React.
Code Explanation
Here's a concise React function using Firebase to add data to Firestore:
import { useState } from 'react';import { database } from '../firebaseConfig';export const addDataToFirebase = async () => { const docRef = database.collection('your-collection').doc(); await docRef.set({ title: 'New post', content: 'Content of the post', createdAt: new Date().toISOString() });}
Breakdown
Importing Dependencies: The function begins by importing
useState
from React (unused in this snippet but typically used for managing state in functional components) anddatabase
from a local Firebase configuration module.Creating a Document Reference: The
addDataToFirebase
function definesdocRef
which points to a new document inside 'your-collection' in Firestore. This is a preparatory step for setting data.Setting Document Data: Using the
set
method of Firestore, the function sets the document with the provided data fields:title
,content
, and a timestampcreatedAt
. Theawait
keyword is used to ensure the operation completes before the function continues or terminates.
Practical Usage
This setup is useful in scenarios where you need to programmatically add data to your Firestore database from a React application. It might be part of a blog management system, user-generated content, or other dynamic content storage solutions.
Conclusion
Using React together with Firebase offers a robust solution for building scalable and dynamic applications. The code provided outlines a foundational approach to interact with Firestore in a React application. To see this code and similar functionalities in action, feel free to explore some of our applications such as high capacity temporary email service, free quality screen recording, and a comprehensive adjective finder.
Original Link: https://dev.to/itselftools/using-react-and-firebase-for-adding-data-to-firestore-2fee

Dev To
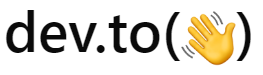
More About this Source Visit Dev To