An Interest In:
Web News this Week
- April 16, 2024
- April 15, 2024
- April 14, 2024
- April 13, 2024
- April 12, 2024
- April 11, 2024
- April 10, 2024
Understanding the useEffect Hook in React
In React, the useEffect hook is a powerful tool that allows you to perform side effects in functional components. It replaces the lifecycle methods used in class components, such as componentDidMount, componentDidUpdate, and componentWillUnmount. In this article, we will explore the useEffect hook and its various use cases.
What is the useEffect Hook?
The useEffect hook is a function provided by React that allows you to perform side effects in a functional component. It takes two parameters: a function that represents the side effect, and an optional array of dependencies. The function inside useEffect is executed after every render by default, but you can control when it runs by specifying the dependencies.
Example 1: Basic usage of useEffect
import React, { useEffect } from 'react';const MyComponent = () => { useEffect(() => { console.log('Component rendered'); }); return ( <div> <h1>Hello, World!</h1> </div> );};export default MyComponent;
In this example, the useEffect hook is used without any dependencies. This means that the side effect function will be executed after every render of the component. In this case, it logs "Component rendered" to the console on each render.
Example 2: Using useEffect with dependencies
import React, { useEffect, useState } from 'react';const MyComponent = () => { const [count, setCount] = useState(0); useEffect(() => { console.log('Count updated:', count); }, [count]); return ( <div> <h1>Count: {count}</h1> <button onClick={() => setCount(count + 1)}>Increment</button> </div> );};export default MyComponent;
In this example, the useEffect hook is used with the count variable as a dependency. It means that the side effect function will only be executed when the count variable changes. In this case, it logs "Count updated" along with the updated count value whenever the button is clicked.
Example 3: Cleanup function in useEffect
import React, { useEffect, useState } from 'react';const MyComponent = () => { const [visible, setVisible] = useState(true); useEffect(() => { console.log('Component mounted'); return () => { console.log('Component unmounted'); }; }, []); return ( <div> {visible ? <h1>Hello, World!</h1> : null} <button onClick={() => setVisible(!visible)}> Toggle Visibility </button> </div> );};export default MyComponent;
In this example, the useEffect hook is used with an empty dependency array, indicating that the side effect function should only run once when the component is mounted. It logs "Component mounted" when the component is mounted and "Component unmounted" when the component is unmounted.
Conclusion:
The useEffect hook is a powerful tool that allows you to handle side effects in React functional components. It offers a simple and concise way to perform actions like fetching data, subscribing to events, or updating the DOM. By understanding the basic usage and various options provided by the useEffect hook, you can effectively manage side effects in your React applications.
Follow me in X/Twitter
Original Link: https://dev.to/ashsajal/understanding-the-useeffect-hook-in-react-knm

Dev To
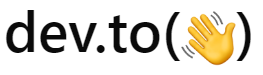
More About this Source Visit Dev To