An Interest In:
Web News this Week
- April 16, 2024
- April 15, 2024
- April 14, 2024
- April 13, 2024
- April 12, 2024
- April 11, 2024
- April 10, 2024
The useRef Hook in React: for Managing References
In React, managing references to DOM elements or values can sometimes be a challenge. However, with the useRef hook, we have a powerful tool at our disposal. useRef allows us to create and maintain a reference to a value or a DOM element throughout the component's lifecycle. In this article, we will explore the useRef hook and its various use cases with practical examples.
Understanding useRef:
The useRef hook is a built-in hook provided by React that returns a mutable ref object. This ref object persists between re-renderings of a component and holds a value that can be accessed and updated without triggering a re-render.
Example 1: Creating a Ref and Accessing the Current Value
import React, { useRef } from 'react';const MyComponent = () => { const inputRef = useRef(null); const handleClick = () => { console.log(inputRef.current.value); }; return ( <div> <input type="text" ref={inputRef} /> <button onClick={handleClick}>Get Value</button> </div> );};
In this example, we create a ref using the useRef hook and assign it to the input element. By accessing the current
property of the ref (inputRef.current
), we can access and log the current value of the input field when the button is clicked.
Example 2: Managing Focus on Input Element
import React, { useRef, useEffect } from 'react';const MyComponent = () => { const inputRef = useRef(null); useEffect(() => { inputRef.current.focus(); }, []); return ( <div> <input type="text" ref={inputRef} /> </div> );};
In this example, we use the useRef hook to create a ref and assign it to the input element. We then use the useEffect hook to focus on the input element when the component mounts. By accessing the current
property of the ref (inputRef.current
), we can call the focus()
method to set the focus on the input element.
Example 3: Storing Previous Values
import React, { useRef, useEffect } from 'react';const MyComponent = () => { const previousCountRef = useRef(0); const count = 5; useEffect(() => { previousCountRef.current = count; }, [count]); return ( <div> <p>Previous Count: {previousCountRef.current}</p> <p>Current Count: {count}</p> </div> );};
In this example, we create a ref using the useRef hook and set its initial value to 0. Inside the useEffect hook, we update the ref's current value to the current count value whenever count changes. This allows us to store and access the previous value of count and display it alongside the current count.
Conclusion:
The useRef hook in React provides a powerful tool for managing references to values and DOM elements. It allows us to persist a value without triggering re-renders and enables us to perform various operations, such as accessing input values, managing focus, and storing previous values. By utilizing the useRef hook effectively, we can enhance the functionality and performance of our React components.
Follow me in X/Twitter
Original Link: https://dev.to/ashsajal/the-useref-hook-in-react-for-managing-references-4cfa

Dev To
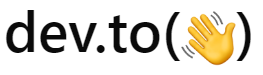
More About this Source Visit Dev To