An Interest In:
Web News this Week
- April 16, 2024
- April 15, 2024
- April 14, 2024
- April 13, 2024
- April 12, 2024
- April 11, 2024
- April 10, 2024
Promices and Async Await
Promises in JavaScript:
Promises are objects representing the eventual completion or failure of an asynchronous operation. They are widely used in JavaScript for handling asynchronous operations such as fetching data from a server, reading files, or any other operations that take time to complete.
Scenario: Fetching Data from an API
Suppose you have an application where you need to fetch data from an API. You can use promises to handle the asynchronous nature of this operation.
// Example function to fetch data from an API using promisesfunction fetchData(url) { return new Promise((resolve, reject) => { fetch(url) .then(response => { if (!response.ok) { throw new Error('Network response was not ok'); } return response.json(); }) .then(data => { resolve(data); }) .catch(error => { reject(error); }); });}// UsagefetchData('https://api.example.com/data') .then(data => { console.log('Data:', data); }) .catch(error => { console.error('Error:', error); });
In this example:
The fetchData function returns a promise that resolves with the fetched data or rejects with an error.
We handle the resolution and rejection of the promise using .then() and .catch() respectively.
Async/Await in JavaScript:
Async/await is a modern approach for writing asynchronous code in a synchronous-looking manner. It provides a syntactic sugar on top of promises, making asynchronous code easier to read and write.
Scenario: Fetching Data from an API Using Async/Await
Let's rewrite the previous example using async/await:
// Example function to fetch data from an API using async/awaitasync function fetchData(url) { try { const response = await fetch(url); if (!response.ok) { throw new Error('Network response was not ok'); } const data = await response.json(); return data; } catch (error) { throw error; }}// Usageasync function getData() { try { const data = await fetchData('https://api.example.com/data'); console.log('Data:', data); } catch (error) { console.error('Error:', error); }}getData();
In this example:
The fetchData function is declared as async, indicating that it returns a promise implicitly.
We use await to pause the execution of the function until the promise returned by fetch resolves.
The getData function is also declared as async, allowing us to use await inside it to wait for the asynchronous operation to complete.
Differences in Industry Example:
Readability and Maintainability:
Promises: While promises provide a good way to handle asynchronous operations, the code can become nested and less readable when dealing with multiple asynchronous calls.
Async/Await: Async/Await simplifies the code structure by making it look more synchronous, thus enhancing readability and maintainability, especially when dealing with complex asynchronous operations.
Error Handling:
Promises: Error handling in promises is done using .catch() at the end of the promise chain, which can lead to scattered error handling logic.
Async/Await: Error handling in async/await is done using try/catch blocks, which makes it easier to handle errors locally within the asynchronous function, leading to more organized error handling.
Sequential Execution:
Promises: Promises are executed sequentially within the promise chain, which can sometimes lead to less intuitive code when dealing with multiple asynchronous operations.
Async/Await: Async/Await allows for a more sequential execution of asynchronous operations, making the code easier to understand and maintain, especially for developers who are more familiar with synchronous programming.****
Original Link: https://dev.to/rohiitbagal/promices-and-async-await-2p0k

Dev To
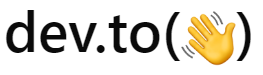
More About this Source Visit Dev To