An Interest In:
Web News this Week
- April 25, 2024
- April 24, 2024
- April 23, 2024
- April 22, 2024
- April 21, 2024
- April 20, 2024
- April 19, 2024
Different possible ways to create objects in JS
In JavaScript, almost "everything" is an object.
Booleans can be objects (if defined with the new keyword)
Numbers can be objects (if defined with the new keyword)
Strings can be objects (if defined with the new keyword)
Dates are always objects
Maths are always objects
Regular expressions are always objects
Arrays are always objects
Functions are always objects
Objects are always objects
All JavaScript values, except primitives, are objects.
Through Object Constructor
const obj= new Object();
This is the simplest way amongst all. This creates and empty object. Currently this approach is not recommended.
Through Object Literal
Also known as object initialiser this is a comma-separated set of name-value pairs wrapped in curly braces.
const obj={ company:"BMW" model:"Z1"}
Through Function Constructor
Create any function and apply the new operator to create object instances. Function name should be capitalised and not camel case.
function ObjectCreate(gender){ this.gender=gender;}const obj= new ObjectCreate("female");
ES6 Syntax
This is a newest way of creating an object.
class ObjectCreate{ constructor(companyName){ this.companyName=companyName; }}const obj= new ObjectCreate("BMW");
Using Singleton pattern
Singleton is a design pattern that tells us that we can create only one instance of a class and that instance can be accessed globally. This is one of the basic types of design pattern. It makes sure that the class acts as a single source of entry for all the consumer components that want to access this state.
A singleton is an object which can only be instantiated one time. Repeated calls to its constructor return the same instance and this helps in ensuring that they don't accidentally create multiple instances.
const singleObj= new(function SingleObject(){ this.year = 2016;})();
References:
Original Link: https://dev.to/shishsingh/different-possible-ways-to-create-objects-in-js-1h74

Dev To
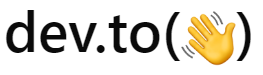
More About this Source Visit Dev To