Playwright Tutorial for Beginners 5 - Performing Actions
Test input
You can easily fill-out form fields with page.fill()
.
page.fill()
focuses on the element and triggers an input
event with the specified text. It works on <input>
, <textarea>
, [contenteditable]
and <label>
associated with an input or text area.
const { test } = require('@playwright/test');test('input text into a test element', async ({ page }) => { await page.goto('https://demoblaze.com'); await page.click('#signin2'); await page.fill('#sign-username', 'zt4ff');});
To view how the test ran, you can set the
headless
andslowMo
property in theplaywright.config.js
file. For instance:
// playwright.config.js// ...use: { launchOptions: { slowMo: 400, headless: false }// ...
page.type()
can also be used but it differs to page.fill()
in sense of it sending a keydown
, keypress/input
and keyup
event for each character of the text while page.fill()
fires only an input
event on the focused element with the specified text.
page.type()
fires every necessary keyboard event and you can even set the delay
of the typing.
Checkboxes and radio buttons
When working with checkboxes and radio buttons, page
provide some methods to check, uncheck and get the state of theses input elements (input[type=checkbox]
, input[type=radio]
or [role=checkbox]
.
// Check the checkboxawait page.check('#agree');// Assert the checked stateexpect(await page.isChecked('#agree')).toBeTruthy();// Uncheck by input <label>.await page.uncheck('#subscribe-label');// Select the radio buttonawait page.check('text=XL');
Selecting options from <select>
// Single selection matching the valueawait page.selectOption('select#colors', 'blue');// Single selection matching the labelawait page.selectOption('select#colors', { label: 'Blue' });// Multiple selected itemsawait page.selectOption('select#colors', ['red', 'green', 'blue']);// Select the option via element handleconst option = await page.$('#best-option');await page.selectOption('select#colors', option);
Using the mouse
You can perform different types of mouse clicks using Playwright. The .click()
method takes an argument of the element you want to click and optional arguments to define the options for the click event. Some examples use cases:
// generic clickingawait page.click('#edit-button');// double clickawait page.dblclick('#edit-button');// right clickawait page.click('#edit-button', { button: 'right' });// using modifiers (like shift, control...) with the click// shift + clickawait page.click('#edit-button', { modifiers: ['Shift'] });// ctrl + clickawait page.click('#edit-button', { modifiers: ['Control'] });// define the position point of click on the elementawait page.click('#edit-button', { position: { x: 0, y: 0 } });
Using .dispatchEvent()
For cases where you want to just trigger events programmatically or trigger a custom event that is not directly implemented in the Playwright API, you can make use of the .dispatchEvent()
method.
You can read about creating and triggering events here
// trigerring a custom event 'build'// .dispatchEvent(selector, type[, eventInit, options])await page.dispatchEvent('#edit-button', 'build')
Original Link: https://dev.to/zt4ff_1/playwright-tutorial-for-beginners-5-performing-actions-4ibd

Dev To
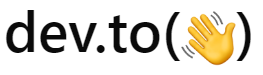
More About this Source Visit Dev To