An Interest In:
Web News this Week
- April 15, 2024
- April 14, 2024
- April 13, 2024
- April 12, 2024
- April 11, 2024
- April 10, 2024
- April 9, 2024
Jenkins Syntax and Logic
Environment Variables
Environment variables are key-value pairs that can be used to store and retrieve data during a Jenkins build. They are often used to store sensitive data, such as API keys or passwords, that should not be hardcoded into your scripts.
Here's an example of how to define an environment variable in Jenkins:
pipeline { agent any environment { MY_VARIABLE = "my value" } stages { stage('Example') { steps { echo "${env.MY_VARIABLE}" } } }}
In this example, we define an environment variable called MY_VARIABLE and give it a value of "my value". We then use the echo command to print out the value of this variable.
Parameters
Parameters allow you to pass data into your Jenkins builds. They are often used to specify different configuration options or to allow users to select options at runtime.
Here's an example of how to define a parameter in Jenkins:
pipeline { agent any parameters { string(name: 'MY_PARAMETER', defaultValue: 'default value', description: 'A description of the parameter') } stages { stage('Example') { steps { echo "${params.MY_PARAMETER}" } } }}
In this example, we define a string parameter called MY_PARAMETER with a default value of "default value" and a description. We then use the echo command to print out the value of this parameter.
Conditions and Logic
Jenkins provides a number of different ways to perform conditional logic and branching within your builds. Here are a few examples:
if statement
The if statement allows you to perform conditional logic within a stage of your pipeline.
pipeline { agent any stages { stage('Example') { steps { if (params.MY_PARAMETER == 'some value') { echo "The parameter is set to 'some value'" } else { echo "The parameter is set to something else" } } } }}
In this example, we use an if statement to check if the value of MY_PARAMETER is equal to "some value". If it is, we print a message indicating that the parameter is set to "some value". If it is not, we print a different message.
when condition
The when condition allows you to control whether a stage should be executed based on some condition.
pipeline { agent any stages { stage('Example') { when { expression { params.MY_PARAMETER == 'some value' } } steps { echo "The parameter is set to 'some value'" } } }}
In this example, we use a when condition to check if the value of MY_PARAMETER is equal to "some value". If it is, we execute the stage and print a message. If it is not, we skip the stage entirely.
Loops
Jenkins also provides a number of ways to perform loops within your pipeline. Here's an example of using a for loop to iterate over a list of items:
pipeline { agent any stages { stage('Example') { steps { def items = ["one", "two", "three"] for (item in items) { echo "Item: ${item}" } } } }}
In this example, we define a list of items and
Original Link: https://dev.to/aws-builders/jenkins-syntax-and-logic-6pm

Dev To
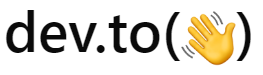
More About this Source Visit Dev To