An Interest In:
Web News this Week
- April 29, 2024
- April 28, 2024
- April 27, 2024
- April 26, 2024
- April 25, 2024
- April 24, 2024
- April 23, 2024
What is prop drilling in React?
Yo ho, my hearties!!!
Prop drilling is the process of passing down data or state through multiple layers of a component hierarchy. By the way, it refers to the practice of passing data from a parent component to its children and then from the children to their own children and so on, until the data reaches the desired component that needs it..
Prop drilling can be a necessary and effective way to manage application state, it can also become a problem when the hierarchy of components becomes too deep or complex. This can lead to several issues. Let's say, code duplication, increased cognitive load and decreased maintainability..
With this article, we will discuss what are props, what prop drilling is and how it can impact your application. We will also explore some best practices for fixing prop drilling in your codebase..
The horizon's embrace, with the wind at our back and the sun on our face. Let's set sail into the depths of prop drilling..
What are Props in React?
Props (aka "Properties") are inputs to components. They are single values or objects containing a set of values that are passed to components on creation similar to HTML tag attributes. Here, the data is passed down from a parent component to a child component.
Props are read-only, meaning that they cannot be modified by the component itself. Used to customize the behavior or appearance of a component and they are passed down through the component tree in a unidirectional flow.
To show you what I mean..
function Snake(props) { return <h1>Hello, {props.name}!</h1>;}function DeadliestSnakes() { return ( <div> <Snake name="Inland Taipan" /> <Snake name="King Cobra" /> <Snake name="Russell's Viper" /> <Snake name="Black Mamba" /> </div> );}
Let's Understand the Prop Drilling
Prop drilling occurs when a parent component passes data down to its children and then those children pass the same data down to their own children. This process can continue indefinitely. At the end, it's a long chain of component dependencies that can be difficult to manage and maintain.
Let's say you have a component that displays a list of blog posts. Each blog post has a title, date, author and content. In order to display this information, the parent component would need to pass down all of this data to its child components and those child components would need to pass it down to their own children and so on..
As you can imagine, this process can become quite complex and unwieldy, especially as your application grows in size and complexity..
The Problems with Prop Drilling
Prop drilling can lead to several issues in your codebase, let's bring it on surface:
Code Duplication
When data needs to be passed down through multiple layers of components, it can result in code duplication. This occurs when the same data is passed down to multiple child components, even though they may not need all of it.
This can lead to bloated and redundant code which can be difficult to maintain and debug..
Increased Cognitive Load
Prop drilling can also increase the cognitive load on developers who need to understand and navigate the component hierarchy. As the number of components and layers increases, it can become difficult to keep track of which components are passing which data and where that data is being used.
This can make it more difficult to identify and fix bugs, as well as to make changes or updates to the codebase.
Decreased Maintainability
Prop drilling can lead to decreased maintainability over time. As your application grows in size and complexity, it can become more difficult to add new features or make changes to existing ones.
At the end, a codebase that is difficult to work with, which can lead to decreased productivity and increased frustration for developers.
A Hunt of Prop Drilling for Cleaner React Code
There are several ways that you can follow to fix prop drilling in your codebase. Let's bring it on surface:
Use a State Management Library
One of the most effective ways to fix prop drilling is to use a state management library, such as Redux or MobX. These libraries provide a centralized store for managing application state which can help to eliminate the need for prop drilling.
Instead of passing data down through multiple layers of components, you can simply connect each component to the store, and access the data that you need directly..
You could refactor your code to use Redux:
- Define a Redux store that holds the list of tasks:
import { createStore } from "redux";const initialState = { snakes: [],};function rootReducer(state = initialState, action) { switch (action.type) { case "ADD_SNAKE": return { ...state, snakes: [...state.snakes, action.payload], }; case "DELETE_SNAKE": return { ...state, snakes: state.snakes.filter((snake) => snake.id !== action.payload.id), }; case "TRACK_SNAKE": return { ...state, snakes: state.snakes.map((snake) => { if (snake.id === action.payload.id) { return { ...snake, tracked: true }; } else { return snake; } }), }; default: return state; }}const store = createStore(rootReducer);
- Connect your components to the Redux store using the
connect()
function:
import { connect } from "react-redux";function SnakeList(props) { const { snakes } = props; return ( <ul> {snakes.map((snake) => ( <Snake key={snake.id} snake={snake} /> ))} </ul> );}function mapStateToProps(state) { return { snakes: state.snakes, };}export default connect(mapStateToProps)(SnakeList);
- Dispatch Redux actions to add, delete or track snakes:
function Snake({ snake, deleteSnake, trackSnake }) { const onTrackSnake = (id) => () => { trackSnake(id); }; const onDeleteSnake = (id) => () => { deleteSnake(id); }; return ( <li> <input type="checkbox" checked={snake.tracked} onChange={onTrackSnake(snake.id)} /> {snake.title} ({snake.tracked ? "Tracked" : "Not Tracked"}) <button onClick={onDeleteSnake(snake.id)}>Delete</button> </li> );}function mapDispatchToProps(dispatch) { return { deleteSnake: (id) => dispatch({ type: "DELETE_TASK", payload: { id } }), trackSnake: (id) => dispatch({ type: "TRACK_SNAKE", payload: { id } }), };}export default connect(null, mapDispatchToProps)(Snake);
Implement Context API
If you don't want to use a full fledged state management library, you can also consider using the Context API in React. This API provides a way to share data between components without the need for prop drilling.
Using the Context API, you can create a context object that holds the data that you need to share. You can then pass this context object down to the child components that need it, without having to pass it down through..
You could use the Context API to share a snake object between components:
- Create a snake context object:
import { createContext } from 'react';export const SnakeContext = createContext({ name: "Black Mamba", fangs: "6.5 mm", speed: "12.5 miles per hour", color: "#964B00", // dark brown});
- Wrap your components in a provider component that provides the snake context:
function Jungle(props) { const snake = { name: "Black Mamba", fangs: "6.5 mm", speed: "12.5 miles per hour", color: "#964B00", // dark brown }; return ( <SnakeContext.Provider value={snake}> <Header /> <Main /> </SnakeContext.Provider> );}
- Use the
useContext()
hook to access the snake object in your child components:
import { useContext } from "react";function Header() { const { color } = useContext(SnakeContext); return ( <header style={{ backgroundColor: color }}> <h1>Snake</h1> </header> );}function Main() { const { name, fangs, speed } = useContext(SnakeContext); return ( <main> <p> Name: <span>{name}</span> </p> <p> Venom Fangs: <span>{fangs}</span> </p> <p> Speed: <span>{speed}</span> </p> </main> );}
Context is not limited to static values. If you pass a different value on the next render, React will update all the components reading it below!!! This is why context is often used in combination with state.
Use cases for context:
- Theming
- Current account
- Managing state
If some information is needed by distant components in different parts of the tree, it's a good indication that context will help you..
In the vast ocean of React development, prop drilling can be a stormy challenge to navigate through.. But my fellow captains, fear not! With the right tools and techniques, you can hoist the sails of clean and efficient code and steer your React application towards smoother waters
Happy coding and smooth sailing on your React adventures!!!
Motivation:
Support
Please consider following and supporting us by subscribing to our channel. Your support is greatly appreciated and will help us continue creating content for you to enjoy. Thank you in advance for your support!
Original Link: https://dev.to/codeofrelevancy/what-is-prop-drilling-in-react-3kol

Dev To
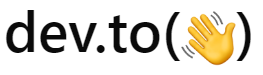
More About this Source Visit Dev To