An Interest In:
Web News this Week
- April 27, 2024
- April 26, 2024
- April 25, 2024
- April 24, 2024
- April 23, 2024
- April 22, 2024
- April 21, 2024
Javascript Object 4
In the last Chapter we've seen a piece of closure called "Lexical Enviroment",Let put that in hold for now and get back into our Object series in this Blog.
In the Begginning Chapter we learnt what is object and how to create it.In the Past Chapter we've created the object using Object literal syntax.Now we are going to create an Object using the CONSTRUCTOR Syntax.
CONSTRUCTOR OBJECT
Before creating the Object,let know about the use cases of Object created using Constructor Syntax,
let person = { firstName: 'Rachael', lastName: 'Ross'};
as you can see this is the basic method of creating an Object using literal Syntax,What If, we wan't to create same Object multiple times but with Different values,here comes the Constructor syntax into use,
The basic Syntax is,
`function Name(firstName, lastName){ this.firstName = firstName; this.lastName = lastName;}`
This is the basic syntax of the Construtor Function,These are as like Normal Function but the rules to call a constructor functions was,
1.The name of a constructor function starts with a capital letter like Person, Document, etc.
2.A constructor function should be called only with the new operator.
Now let's create an Object with our Constructor Function,
In order to create a new Object,we need to call our Function "New" keyword to create a new instance of an Object
For Example,
`let friends = new Name('Chandler','Monica');`
That's it very quite right,Then what's go behind this while Creating the new Objects.
1.Create a new empty object and assign it to the this variable.
2.Assign the arguments 'Chandler' and 'Monica' to the firstName and lastName properties of the object.
3.Return the 'this' value.
So,this might be equivalent to the
`
`
function Name(firstName, lastName) {
// this = {};
// add properties to thisthis.firstName = firstName;this.lastName = lastName;// return this;
`
`
Pretty Straigt.We can also add method to the Constructor function,
let's create a method to get the fullname of an Object,as follows.
`function Name(firstName, lastName) { this.firstName = firstName; this.lastName = lastName; this.getFullName = function () { return this.firstName + " " + this.lastName; };}`
Now we've added a method to our function.Let's call it out
`
`
let friends = new Name('Ross','Rachael');
console.log(person.getFullName());
Output:
Ross Rachael
`
`
Simple but not efficient,because whenever you create a new instance for every Object getFullName() will be duplicated and it is not memory efficient.Inorder to overcome this,we can create this method in the Object "Prototype" so that every instance of that type can use the method.
what if we use return inside the constructor function,
Returning from constructor functions
Typically, a constructor function implicitly returns this that set to the newly created object. But if it has a return statement, then heres are the rules:
1.If return is called with an object, the constructor function returns that object instead of this.
2.If return is called with a value other than an object, it is ignored.
That's all about Constructor Function now,we'll see more about this in upcoming post.
Many Thanks for Your Time,
Sam
Original Link: https://dev.to/samr/javascript-object-4-26n7

Dev To
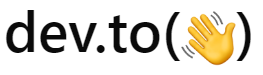
More About this Source Visit Dev To