An Interest In:
Web News this Week
- April 3, 2024
- April 2, 2024
- April 1, 2024
- March 31, 2024
- March 30, 2024
- March 29, 2024
- March 28, 2024
Design Pattern: Strategy (TS)
The strategy pattern is useful in cases where we have multiple algorithms or strategies that can be interchangeable, and we want to encapsulate them behind a common interface. This allows us to easily switch between different strategies without changing the client code, and also promotes separation of concerns by keeping the algorithms isolated in their own classes.
This pattern can be particularly useful in scenarios where the behavior of an object needs to vary dynamically based on different conditions or inputs. It provides a flexible and extensible way to encapsulate different algorithms or strategies and allows for easy customization and modification without modifying the core logic of the client code.
interface SortStrategy { sort(data: number[]): number[];}class BubbleSortStrategy implements SortStrategy { sort(data: number[]): number[] { // Implementation of bubble sort algorithm console.log("Sorting using bubble sort strategy"); // ... return data; }}class QuickSortStrategy implements SortStrategy { sort(data: number[]): number[] { // Implementation of quick sort algorithm console.log("Sorting using quick sort strategy"); // ... return data; }}class SortContext { private strategy: SortStrategy; constructor(strategy: SortStrategy) { this.strategy = strategy; } setStrategy(strategy: SortStrategy): void { this.strategy = strategy; } sort(data: number[]): number[] { return this.strategy.sort(data); }}// Usage exampleconst data = [5, 2, 9, 1, 5, 6];const bubbleSort = new BubbleSortStrategy();const quickSort = new QuickSortStrategy();const context = new SortContext(bubbleSort);context.sort(data); // Sorting using bubble sort strategycontext.setStrategy(quickSort);context.sort(data); // Sorting using quick sort strategy
In this example, the strategy pattern is used to encapsulate different sorting algorithms (bubble sort and quick sort) behind a common SortStrategy interface, and the client can switch between them dynamically at runtime using the SortContext class. This provides flexibility and extensibility in choosing different sorting strategies without changing the core logic of the client code. It also promotes separation of concerns by keeping the sorting algorithms isolated in their own classes, making the code more maintainable and testable.
The strategy pattern is commonly used in situations where different algorithms need to be applied to the same problem or input data, and the choice of algorithm needs to be made at runtime based on specific conditions or configuration settings.
Original Link: https://dev.to/daniyarotynshin/design-pattern-strategy-ts-3e55

Dev To
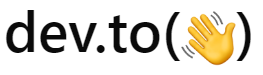
More About this Source Visit Dev To