An Interest In:
Web News this Week
- April 27, 2024
- April 26, 2024
- April 25, 2024
- April 24, 2024
- April 23, 2024
- April 22, 2024
- April 21, 2024
April 4, 2023 08:44 pm GMT
Original Link: https://dev.to/jgamaraalv/react-render-props-pattern-314j
[React] Render Props Pattern
What is render props pattern? Understand this powerful React pattern.
With the Render Props pattern, we pass components as props to other components.
The components that are passed as props can, in turn, receive other props from that parent component.
Render props make it easy to reuse logic across multiple components.
Implementation
If we wanted to implement an input with which a user can convert a Celsius temperature to Kelvin and Fahrenheit, we can use the renderKelvin and renderFahrenheit render props.
These props receive the input value, which they convert to the correct temperature in K or F.
function Input(props) { const [value, setValue] = useState(""); return ( <> <input value={value} onChange={(e) => setValue(e.target.value)} /> {props.renderKelvin({ value: value + 273.15 })} {props.renderFahrenheit({ value: (value * 9) / 5 + 32 })} </> );}export default function App() { return ( <Input renderKelvin={({ value }) => <div className="temp">{value}K</div>} renderFahrenheit={({ value }) => <div className="temp">{value}F</div>} /> );}
Benefits
- Reuse: Since render props can be different every time, we can make components that receive render props highly reusable for multiple use cases.
- Separation of concerns: We can separate the logic of our application from the rendering components through render props. The stateful component that receives a render prop can pass the data to stateless components, which only render the data.
- Solution to HOC problems: Since we pass props explicitly, we solve the problem of implicit props from HOCs. The props that need to be passed to the element are all visible in the render prop's argument list. We know exactly where certain props come from.
Drawbacks
- Not as necessary with hooks: Hooks have changed the way we can add reuse and data sharing to components, which can replace the render props pattern in many cases. However, there are still cases where the render props pattern can be the best solution.
Comment below if you knew about this pattern and if you use it in your daily work!
Liked it? Check out other articles written by me:
Understanding closures
Understanding classes and prototypes
Understanding Higher Order Function
Original Link: https://dev.to/jgamaraalv/react-render-props-pattern-314j
Share this article:
Tweet
View Full Article

Dev To
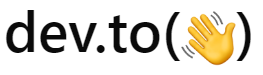
More About this Source Visit Dev To