An Interest In:
Web News this Week
- April 26, 2024
- April 25, 2024
- April 24, 2024
- April 23, 2024
- April 22, 2024
- April 21, 2024
- April 20, 2024
React Components 101: Building Reusable Components
Original Blog Post : Link
React is a popular JavaScript library for building user interfaces, and one of its key features is the ability to create reusable components. In this blog post, we'll go over the basics of creating and using components in a React application.
What are React Components?
In React, a component is a piece of code that represents a part of a user interface. For example, you might have a component that represents a button, or a component that represents a form. Components allow you to split your UI into smaller, reusable pieces that can be easily managed and maintained.
There are two types of components in React: functional components and class-based components. Functional components are simple JavaScript functions that accept props (short for "properties") as an argument and return a React element. Class-based components are JavaScript classes that extend the React.Component class and have a render method that returns a React element.
Creating a React Component
To create a functional component, you just need to define a function that returns a React element. For example, here's a functional component that represents a button:
function Button(props) { return ( <button>{props.children}</button> );}
To create a class-based component, you need to define a class that extends React.Component and has a render method. For example, here's a class-based component that represents a form:
class Form extends React.Component { render() { return ( <form> {this.props.children} </form> ); }}
Using Props
Props are a way to pass data from a parent component to a child component. In the examples above, the Button and Form components accept props as an argument and use them to render dynamic content.
For example, you can use the Button component like this:
<Button>Click me!</Button>
This will render a button with the text "Click me!" inside of it.
You can also pass props to a component using the JSX syntax. For example, you can pass a "type" prop to the Button component like this:
<Button type="submit">Submit</Button>
This will render a button with the type "submit", which can be used to submit a form.
Using State
In addition to props, components can also have state, which is a way to store and manage data that affects a component's behavior. State is only available in class-based components, and it should be used sparingly, as it can make a component difficult to reason about if it's used excessively.
To use state in a class-based component, you need to define a constructor and set the initial state. For example:
class Form extends React.Component { constructor(props) { super(props); this.state = { name: '', email: '', message: '' }; } render() { return ( <form> <input type="text" value={this.state.name} onChange={event => this.setState({ name: event.target.value })} /> <input type="email" value={this.state.email} onChange={event => this.setState({ email: event.target.value })} /> <textarea value={this.state.message} onChange={event => this.setState({ message: event.target.value })} /> </form> ); }}
Remember, the key to building effective components is to keep them independent and focused on a single task, and to use props and state wisely to manage their behavior. By following these principles, you can create a clean and maintainable codebase that is easy to understand and debug.
Conclusion
I hope this helps! If you have any questions or comments, feel free to leave them below. Happy coding!
Thank You for reading till here. Meanwhile you can check out my other blog posts and visit my Github and my socials here .
I am currently working on DVS Tech Labs
This article was created with the help of AI
Original Link: https://dev.to/devarshishimpi/react-components-101-building-reusable-components-3pf9

Dev To
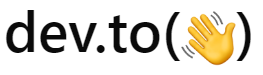
More About this Source Visit Dev To