An Interest In:
Web News this Week
- April 2, 2024
- April 1, 2024
- March 31, 2024
- March 30, 2024
- March 29, 2024
- March 28, 2024
- March 27, 2024
JavaScript Primitive vs Reference Values
Introduction:
JavaScript has mainly 2 types of values, namely
- Primitive values (atomic values)
- Reference values (objects which might consist of multiple kinds of values).
In this article, we will learn about
- Memory in JavaScript
- Types of Values in JavaScript
- Properties of Primitive and Dynamic values
- Copying property
Stack and Heap Memory:
Any variable in JS is stored in either heap or stack.
Static data:
It is the type of data whose size is known and so is fixed at compile time. It includes
- Primitive values: null, undefined, Boolean, number, string, symbol, and BigInt
- Reference values: Variables that are references and point to other objects
The above two have fixed sizes and so they are stored in the stack.
For example, the following declares two variables and initializes their values to a literal string and a number:
let name = 'John';let age = 25;
Since the above two are primitive values, they will be stored in stack memory as shown below
Image credits:JavaScript Tutorial
Note: Many languages consider strings as an object, but JS considers strings as a primitive value
JavaScript stores objects, and functions in heap. The JS engine doesn't allocate fixed space for these, the memory is expanded as it is used.
Eg.
let name = 'John';let age = 25;let person = { name: 'John', age: 25,};
Image credits:JavaScript Tutorial
Here, the 3 variables, name, age, and person(a reference that refers to the object in the heap) are stored in the stack. The actual values of objects are stored in a heap.
Dynamic properties:
A reference value can be used to add, change or delete properties at any time. However, this is not the same case for primitive values. In JS, syntactically primitive values are allowed to add properties, but they won't have any effects and are not saved.
Image credits:JavaScript Tutorial
Let us see the same example in the case of primitive values
Image credits:JavaScript Tutorial
How copying values work:
Primitive values:
In the case of primitive values, when copy statement is executed, the JS engine creates a copy of the value and assigns that value to the new variable.
Eg.
let age = 25;let newAge = age;
Here,
- First, age is initialized with the value 25.
- Second, when the newAge variable is assigned with age, the JS engine creates a copy of the value of the age variable (creates a copy of value 25) and then assigns it to newAge.
Image credits:JavaScript Tutorial
So change in one place doesn't affect the change in another.
Reference Values:
When you assign a reference value from one variable to another, the JavaScript engine creates a reference so that both variables refer to the same object on the heap memory. This means that if you change one variable, itll affect the other.
let person = { name: 'John', age: 25,};let member = person;member.age = 26;console.log(person);console.log(member);
Here both of them give the same output because the "member" reference value is pointing to the same object in the heap.
Image credits:JavaScript Tutorial
Summary:
- Javascript has two types of values: primitive values and reference values.
- You can add, change, or delete properties to a reference value, whereas you cannot do it with a primitive value.
- Copying a primitive value from one variable to another creates a separate value copy. It means that changing the value in one variable does not affect the other.
- Copying a reference from one variable to another creates a reference so that two variables refer to the same object. This means that changing the object via one variable reflects in another variable.
Original Link: https://dev.to/indirakumar/javascript-primitive-vs-reference-values-1kmm

Dev To
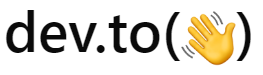
More About this Source Visit Dev To