An Interest In:
Web News this Week
- April 27, 2024
- April 26, 2024
- April 25, 2024
- April 24, 2024
- April 23, 2024
- April 22, 2024
- April 21, 2024
Fetch vs Axios in Javascript
Hello there ! In this blog, we are going to learn how to implement APIs by using Fetch API and Axios
Before we get started, the only pre-requisite needed for this tutorial is basic understanding of javascript.
Table of contents
- What is fetch?
- Syntax of fetch
- What is axios?
- Syntax for axios
- Key differences b/w fetch and axios
What is Fetch ?
Fetch is a standard way of sending asynchronous api calls to the server in web applications. It is a feature of ECMASCRIPT6(ES6) and was introduced back in 2015.
The Fetch API provides a method called fetch () which is defined on the window object. It needs an argument which is the url of the endpoint (resource). This method returns a promise which is resolved to retrieve the response.
Let us see an example, where we are fetching some data from an endpoint
const fetchNames = ()=>{ fetch("https://localhost:5000/api/names/") .then((res)=> res.json()) // successful req, change to json .then((data)=>{ console.log(data); // data contains the json object }) .catch(error=>{ console.log(error); // something went wrong })}
Note that, for Post request we also need to include the options
For example, we are sending a name with id to the server
const sendName=()=>{ fetch("http://localhost:5000/api/user/", { // the method type method : 'POST', // contents to send i.e., the body body: JSON.stringify({ name : 'David', id:"1" }), // headers of the request headers:{ 'Content-type':'Application/json' } }) .then( res => res.json()) .then( data=> console.log(data)) .catch(error => console.log(error))}
So, we know how fetch works. Now, let us see axios.
What is Axios?
Axios is a javascript library used to make HTTP requests from node js(runtime for javascript) or XMLHttpRequests (XHR) from the browser. This too supports the promise api that is native to ES6.
Example for showing how we can fetch data and post data using axios
// get request const fetchNames = async()=>{ // we are using asynchronous function so we need to await for the req to be processed try{ const response = await axios.get('http://localhost:5000/api/names/'); console.log(response.data) }catch(error){ console.log(error); }}// post requestconst postName = async()=>{ // same as previous try{ const response = await axios.post('http://localhost:5000/api/users/',{ name:'David', id:1 }); // log the data from the response console.log(response.data); }catch(error){ console.log(error); // something went wrong! }}
Key differences between fetch and axios
Fetch | Axios |
---|---|
Fetch is built-in for most modern web browsers, so no installation is required | Axios is a stand-alone third party package (library) which requires installation |
Fetch has no url in the request object | Axios has url in it's request object |
It doesn't have XSRF (cross-site request forgery) protection | It has XSRF protection in-built |
Request is made then the response is converted to json | Here, the response is automatically transformed to json |
Request has body which is converted to string | Request has data which contains the object itself |
Cannot abort requests | Has request-timeouts for cancelling requests |
Hopefully, I have been able to clear the methods of making api calls to the server. If you have understood, then like the post, comment and share so that others can benifit from this.
Thank you! Keep developing !
Original Link: https://dev.to/suman373_30/fetch-vs-axios-in-javascript-4oj0

Dev To
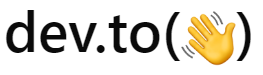
More About this Source Visit Dev To