An Interest In:
Web News this Week
- April 22, 2024
- April 21, 2024
- April 20, 2024
- April 19, 2024
- April 18, 2024
- April 17, 2024
- April 16, 2024
The easiest way to send emails from the server
Web sites and applications allow for communication between service providers and their clients. You have probably noticed almost every website has a contact form. In this tutorial, am showing you the simplest way to send emails over a NodeJS backend so you can soon implement it for your clients or on your personal website using the Nodemailer module.
1. What do we need?
You need to have NodeJS installed, if you don't, you can download it from this link
2. What is Nodemailer?
From the official website
Nodemailer is a module for Node.js applications to allow easy-as-cake email sending.
The project got started back in 2010 when there was no sane option to send email messages, today it is the solution most Node.js users turn to by default
3. How to set up Nodemailer?
To install nodemailer, we use npm
(node package manager). npm
is automatically installed with NodeJS.
Run the code below in your command prompt
npm install nodemailer
When it's done downloading, it can now be included in your application as below
const nodemailer = require("nodemailer");
3. How to send an email?
- First, you need to set up a transporter, this is going to be an object that is able to send mails
- Secondly, you need to set up your mailData, you can call it anything else, it is an object that defines the mail content, read here for more information
- Lastly, we need to call the tell the transporter to send our mailData.Easy
4. Easy example
The example below shows how to use your Gmail account to send an email.
const nodemailer = require('nodemailer');const transporter = nodemailer.createTransport({ service: 'gmail', auth: { user: '[email protected]', pass: 'your_app_password' }});const mailData = { from: '[email protected]', to: '[email protected]', subject: 'Sending Email using Node.js', text: 'That was easy!'};transporter.sendMail(mailData, (error, info) => { if (error) { console.log(error); } else { console.log('Email sent: ' + info.response); }});
The
pass
app password is generated from Gmail, if you don't know how to generate the app password, watch this 2-minute video to guide you.
5. How to send to many recipients at once?
Many times there is a need to send the email to many people, maybe the Boss and other team members.
To send an email to more than one receiver, add them to the "to" property of the mailData object, separated by commas as shown below:
Simple example: Sending to multiple recipients.
const mailData = { from: '[email protected]', to: '[email protected], [email protected]', subject: 'Sending Email using Node.js', text: 'That was easy!'}
6. How to send HTML?
I used to receive well-designed HTML pages from those big organizations, if you've received them too and been wondering how they do it, here you go.
To send HTML formatted text in your email, use the "html" property instead of the "text" property as shown below:
Simple example: Sending HTML
var mailData = { from: '[email protected]', to: '[email protected]', subject: 'Sending Email using Node.js', html: '<h1>Welcome</h1><p>That was easy!</p>'}
If you found this little content use, consider subscribing so that you do not miss out on my upcoming articles, thank you so much.
Original Link: https://dev.to/jumashafara/the-easiest-way-to-send-emails-from-the-server-4gfm

Dev To
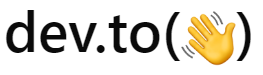
More About this Source Visit Dev To