An Interest In:
Web News this Week
- April 2, 2024
- April 1, 2024
- March 31, 2024
- March 30, 2024
- March 29, 2024
- March 28, 2024
- March 27, 2024
August 26, 2022 03:56 pm GMT
Original Link: https://dev.to/shubhamtiwari909/splice-method-in-javascript-5e9p
Splice method in javascript
Hello Guys today i will be discussing about splice array method in javascript .
Let's get started...
What is splice() ?
- splice method is used to remove all or any number of elements from any index in array, insert any number of elements at any index in array, replace elements at any index with any number of elements.
- The main thing is that it makes changes to original array so be careful while using this method.
- I will explain it with some examples.
Syntax -
splice(startIndex,deleteNumber,item1,item2,.....itemN);
- startIndex - it is the index number where we will perform the operation of splice for adding ,deleting ,replacing.It can be negative numbers as well like negative indexing.
- deleteNumber - it is the count of elements which will be deleted like if it is set to 2 , then 2 items from startIndex number will be deleted including the startIndex.
- items - it is those items which will be added or replaced and it could be anything like number, string, array, boolean, object, etc.
Example 1 -
const array1 = [1,2,3,4,5];// remove all elements starting from index 2(inclusive)const removeAll = array1.splice(2);// output - [1,2]
Example 2 -
const array1 = [1,2,3,4,5];// remove two elements starting from index 1 (inclusive)const removeTwo = array1.splice(1,2)// output - [1,4,5]
Example 3 -
const array1 = [1,2,3,4,5];// remove 0 elements and insert two elements after index 2const removeAndInsert = array1.splice(2,0,99,100)// output - [1,2,99,100,3,4,5]
Example 4 -
const array1 = [1,2,3,4,5];// remove two elements and insert four elements after index 2const removeTwoAndInsert = array1.splice(2,2,101,102,103,104);// output - [1,2,101,102,103,104,5]
Example 5 -
const array1 = [1,2,3,4,5];// remove all elements from negative Index -2 means 2nd element from lastconst negativeIndexing = array1.splice(-2)// [1,2,3]
Example 6 -
const array1 = [1,2,3,4,5];// remove one element from negative Index -2 // means 2nd element from last and insert 3 elements thereconst negativeIndexingRemove = array1.splice(-2,1,10,11,12)// output - [1,2,3,10,11,12,5]
Example 7 -
const array1 = [1,2,3,4,5];// insert anything at the end of the arrayconst anything = array1.splice(array1.length,0,"javascript",true,false,undefined,null,[6,7,8,9],{name:"shubham",age:21},[[10,11],[12,13]])// output - [1,2,3,4,5,'javascript',true,false,undefined,null,// [6,7,8,9],{name:"shubham",age:21},[[10,11],[12,13]]]
Example 8 -
const array1 = [1,2,3,4,5];// if we try to change the values inside function// it will still change the original array const changeArray = (arr) => { return arr.splice(1,2)}changeArray(array1)// output - [1,4,5]
THANK YOU FOR CHECKING THIS POST
You can contact me on -
Instagram - https://www.instagram.com/s_h.u_b.h_a.m_2k99/
LinkedIn - https://www.linkedin.com/in/shubham-tiwari-b7544b193/
Email - [email protected]
^^You can help me by some donation at the link below Thank you ^^
--> https://www.buymeacoffee.com/waaduheck <--
Also check these posts as well
https://dev.to/shubhamtiwari909/js-push-and-pop-with-arrays-33a2/edit
https://dev.to/shubhamtiwari909/tostring-in-js-27b
https://dev.to/shubhamtiwari909/join-in-javascript-4050
https://dev.to/shubhamtiwari909/going-deep-in-array-sort-js-2n90
Original Link: https://dev.to/shubhamtiwari909/splice-method-in-javascript-5e9p
Share this article:
Tweet
View Full Article

Dev To
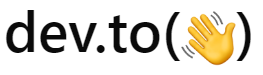
More About this Source Visit Dev To