An Interest In:
Web News this Week
- April 26, 2024
- April 25, 2024
- April 24, 2024
- April 23, 2024
- April 22, 2024
- April 21, 2024
- April 20, 2024
Rest Parameters & Spread Syntax
Hi there! If you're looking to learn or just revise the REST Parameters & SPREAD syntax, then this blog is for you. We'll unfold both of the JavaScript concepts in a simple, easy to understand manner.
Let's Start with REST PARAMETERS
Imagine you're writing a function for adding two numbers in JavaScript. It would look something like this
function addTheNumbers (numberOne, numberTwo) { return numberOne + numberTwo; } // PRINTING THE VALUES console.log(addTheNumbers(2,3)); // expected output : 5
Works fine, yes! Now what if someone asks you to create a function for adding 5 numbers. It won't be convenient to manually add the numbers parameter till numerFive.
This is where REST parameters comes handy....
Let's quickly jump into how to use them. The REST parameter can be included in the function definition by using three dots ...
followed by the name of the array that will contain them.
function addTheNumbers (...args) { let sum = 0; for (let arg of args){ sum = sum + arg } return sum } // PRINTING THE VALUES console.log(addTheNumbers(1,2,3,4,1)); //expected output : 11
The dots literally mean gather the remaining parameters into an array.
Important note - The REST parameters must be at the end
Below is an Incorrect way of using REST parameters
function f(arg1, ...rest, arg2) { // arg2 after ...rest is incorrect}
Let's look into one more example
const funcNames = (name, surname, ...args) => { console.log(name, surname, args);}funcNames("Raj", "Bhinde", "Dhaval","Bhinde")
Output - Raj Bhinde [ 'Dhaval', 'Bhinde' ]
Here, we saw that it took the name, surname & then condensed all the other parameters in one array. You can also access the index of these arrays using args[0]
Now before we move onto Spread syntax, considering the above examples let's keep in mind that REST syntax collects multiple elements and "condenses" them into a single element as we saw above.
SPREAD is opposite of rest syntax. Although the syntax looks the same, they come with slightly different semantics.
Before telling you what Spread does, we'll observe with the help of an example what SPREAD does-
Let's say we have a function which multiplies the numbers passed in arguments
const muliplyTheNumbers = (x,y,z) => { return x*y*z}console.log(muliplyTheNumbers(1,2,3)); //expected output : 6
This would work perfectly fine, but now let's say we have an array of numbers that we would like to multiply
const arrayOfNumbers = [2,3,5,2,2];
If you now pass the array in the function call it would give you a NaN. That is because the function expects a list of numeric arguments & not a single array.
Here's when Spread syntax comes to rescue!
console.log(muliplyTheNumbers(...arrayOfNumbers));
When ...arrayOfNumbers is used in the function call, it expands an iterable object arrayOfNumbers into the list of arguments.
We can also pass multiple arrays with the help of Spread syntax
const arrayOfNumbersTwo = [10,2];
can be passed as muliplyTheNumbers(...arrayOfNumbers,...arrayOfNumbersTwo);
& is absolutely valid. We can also add normal values at the start/inbetween/end of function call.
Spread syntax can also be used to merge two arrays
const arr1 = ["1","2","3"];const arr2 = ["4","5"]console.log([...arr1,...arr2]); output: ['1', '2', '3', '4', '5']console.log(...arr1,...arr2); output : 1 2 3 4 5
In the above examples we used an array, but spread works with any iterable.
let stringValue = "TheBoys";console.log([...stringValue]); output : ['T', 'h', 'e', 'B', 'o', 'y', 's']
It can also be used to clone an object like this -
const obj1 = { firstName : "Raj"}const obj2 = { ...obj1, surname : "Bhinde"}console.log(obj2);// expected output : { firstName: 'Raj', surname: 'Bhinde' }
Similarly, we can merge two objects as well.
So, that was all folks! Thank you for reading so far. I hope it was a good read for you.
Here's a quick summary to not forget the difference between REST & SPREAD
REST OPERATOR -
- The REST operator is known for de-structuring of elements, then it collects the leftover element to make it an array.
- REST Operator must be at the end of the arguments else it doesn't work
SPREAD OPERATOR -
- SPREAD Operator extracts the collected elements to a single element
- SPREAD can be used anywhere irrespective of its position.
Original Link: https://dev.to/irajbhinde/rest-parameters-spread-syntax-2k92

Dev To
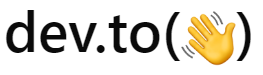
More About this Source Visit Dev To