An Interest In:
Web News this Week
- April 2, 2024
- April 1, 2024
- March 31, 2024
- March 30, 2024
- March 29, 2024
- March 28, 2024
- March 27, 2024
How to Build a Connector Map in JS
Connector maps are designed to be ideal for visualizing routes and other links between locations in geospatial data analysis. In this tutorial, youll learn how to quickly create a compelling interactive one using JavaScript.
Step by step, we will be visualizing a route of the famous ancient Silk Road. Well start with the development of a basic JS connector map in four moves and then make a few tweaks to make it look awesome. Lets start the voyage!
Connector Map Preview
Here is a preview of how the final JavaScript-based connector map of the tutorial will look.
Without more ado, lets get to the connector mapping business!
Building a Basic JS Connector Map
The logic behind creating connector maps with JavaScript is pretty straightforward. The entire path can be broken down into four fundamental moves:
- Create an HTML page.
- Add the necessary JavaScript files.
- Prepare and load the data.
- Write some JS code to draw the connector map.
1. Create an HTML page
First of all, where should we put our interactive connector map? Lets create a basic HTML page.
Right there, we add an HTML block element and assign it a unique identifier. Then, we set a style declaration in a style sheet.
<!DOCTYPE html><html lang="en"> <head> <meta charset="utf-8"> <title>JavaScript Connector Map</title> <style type="text/css"> html, body, #container { width: 100%; height: 100%; margin: 0; padding: 0; } </style> </head> <body> <div id="container"></div> </body></html>
In the example above, the block element is <div>
. Its id
attribute is set as container. The width
and height
parameters of the element are 100%, which will ensure the connector map is displayed over the entire screen.
2. Add the necessary JavaScript files
Second, we need to reference all scripts that will be used for data visualization, in the <head>
section.
In most cases, you can choose from a wide range of JavaScript charting libraries to handle interactive data visualization on the web. Each has pros and cons, so which one to pick always depends on exactly what, where, and how you want. The basic approach is quite similar for all, though. In this tutorial, for illustration, we will be using a JS charting library called AnyChart. It has connector maps among the supported chart types, a detailed documentation, and a free version.
We need the Core and Geo Map modules to take care of data visualization in the form of a connector map, geodata for a world map, and the Proj4js library to take care of geographic coordinates.
<!DOCTYPE html><html lang="en"> <head> <meta charset="utf-8"> <title>JavaScript Connector Map</title> <script src="https://cdn.anychart.com/releases/8.11.0/js/anychart-core.min.js"></script> <script src="https://cdn.anychart.com/releases/8.11.0/js/anychart-map.min.js"></script> <script src="https://cdn.anychart.com/releases/8.11.0/geodata/custom/world/world.js"></script> <script src="https://cdnjs.cloudflare.com/ajax/libs/proj4js/2.8.0/proj4.js"></script> <style type="text/css"> html, body, #container { width: 100%; height: 100%; margin: 0; padding: 0; } </style> </head> <body> <div id="container"></div> <script> // The JS connector map code will be written here. </script> </body></html>
3. Prepare and load the data
Third, lets set data for our JavaScript-based connector map.
The Silk Road was a series of routes. Lets visualize one of them, from Xian to Venice, which lied through the cities of Lanzhou, Dunhuang, Qiemo, Hotan, Kashgar, Merv, Tehran, Baghdad, Antioch, and Athens.
In a connector map, the start and end points of each connection line are defined using their latitude and longitude. In order to get the coordinates of the cities mentioned above, we can use one of multiple coordinate generator tools. I have already collated them from LatLong.net. Here is the list of the cities along the route that will be visualized, with their latitudes and longitudes:
In a connector map, the start and end points of each connection line are defined using their latitude and longitude. In order to get the coordinates of the cities mentioned above, we can use one of multiple coordinate generator tools. I have already collated them from LatLong.net. Here is the list of the cities along the route that will be visualized, with their latitudes and longitudes:
City | Latitude, longitude |
---|---|
Xian | 33.62,113.34 |
Lanzhou | 36.05,103.79 |
Dunhuang | 40.14, 94.66 |
Qiemo | 38.14, 85.52 |
Hotan | 37.11, 79.91 |
Kashgar | 39.46, 75.99 |
Merv | 37.66, 62.16 |
Tehran | 35.68, 51.38 |
Baghdad | 33.31, 44.36 |
Antioch | 36.19, 36.16 |
Athens | 37.98, 23.72 |
Venice | 45.44, 12.31 |
For a connector map, data can be arranged as objects or arrays. We will be using the object notation in this tutorial. So, our dataset will be an array of objects, where each object is a connector defined by four values: the latitude of the start point, the longitude of the start point, the latitude of the end point, and the longitude of the end point. Heres a dataset for our connector map visualization:
var dataSet = [ {points: [33.62, 113.34, 36.05, 103.79]}, {points: [36.05, 103.79, 40.14, 94.66]}, {points: [40.14, 94.66, 38.14, 85.52]}, {points: [38.14, 85.52, 37.11, 79.91]}, {points: [37.11, 79.91, 39.46, 75.99]}, {points: [39.46, 75.99, 37.66, 62.16]}, {points: [37.66, 62.16, 35.68, 51.38]}, {points: [35.68, 51.38, 33.31, 44.36]}, {points: [33.31, 44.36, 36.19, 36.16]}, {points: [36.19, 36.16, 37.98, 23.72]}, {points: [37.98, 23.72, 45.44, 12.31]}];
Now, just a few lines of JS code to get our connector map up and running on the web page!
4. Write some JS code to draw the connector map
Fourth and finally, lets put together the JavaScript code that will draw the connector map.
At the start, we add the anychart.onDocumentReady()
function, which will enclose all the JS connector mapping code. This makes sure that everything inside it will execute only after the HTML page is loaded.
<script> anychart.onDocumentReady(function () { // The connector map code will be written here. });</script>
So, we add the data just prepared in the third step.
anychart.onDocumentReady(function () { var dataSet = [ {points: [33.62, 113.34, 36.05, 103.79]}, {points: [36.05, 103.79, 40.14, 94.66]}, {points: [40.14, 94.66, 38.14, 85.52]}, {points: [38.14, 85.52, 37.11, 79.91]}, {points: [37.11, 79.91, 39.46, 75.99]}, {points: [39.46, 75.99, 37.66, 62.16]}, {points: [37.66, 62.16, 35.68, 51.38]}, {points: [35.68, 51.38, 33.31, 44.36]}, {points: [33.31, 44.36, 36.19, 36.16]}, {points: [36.19, 36.16, 37.98, 23.72]}, {points: [37.98, 23.72, 45.44, 12.31]} ];});
Everything else goes into the same enclosing function. We create a map using the map()
function, then a connector map series using the connector()
function (and passing the dataset into it), and set the world map geodata.
var map = anychart.map();var series = map.connector(dataSet);map.geoData(anychart.maps['world']);
Then we can add a title to make it clear what is shown on the map.
map.title("Silk Road Trade Route from Xian to Venice");
And the last two quick lines will place the map within the ontainer element and display it on the page.
map.container('container');map.draw();
There you go! Our connector map is ready, and it has been quite easy to make it in just a few lines of code!
The interactive version of this basic JavaScript connector map can be found on JSFiddle [and on AnyChart Playground] where you can review the code and play around with it. I am also putting the full code below.
<!DOCTYPE html><html lang="en"> <head> <meta charset="utf-8"> <title>JavaScript Connector Map</title> <script src="https://cdn.anychart.com/releases/8.11.0/js/anychart-core.min.js"></script> <script src="https://cdn.anychart.com/releases/8.11.0/js/anychart-map.min.js"></script> <script src="https://cdn.anychart.com/releases/8.11.0/geodata/custom/world/world.js"></script> <script src="https://cdnjs.cloudflare.com/ajax/libs/proj4js/2.8.0/proj4.js"></script> <style type="text/css"> html, body, #container { width: 100%; height: 100%; margin: 0; padding: 0; } </style> </head> <body> <div id="container"></div> <script> anychart.onDocumentReady(function () { // create a data set for connectors var dataSet = [ {points: [33.62, 113.34, 36.05, 103.79]}, {points: [36.05, 103.79, 40.14, 94.66]}, {points: [40.14, 94.66, 38.14, 85.52]}, {points: [38.14, 85.52, 37.11, 79.91]}, {points: [37.11, 79.91, 39.46, 75.99]}, {points: [39.46, 75.99, 37.66, 62.16]}, {points: [37.66, 62.16, 35.68, 51.38]}, {points: [35.68, 51.38, 33.31, 44.36]}, {points: [33.31, 44.36, 36.19, 36.16]}, {points: [36.19, 36.16, 37.98, 23.72]}, {points: [37.98, 23.72, 45.44, 12.31]} ]; // create a map var map = anychart.map(); // create a connector series var series = map.connector(dataSet); // set geodata map.geoData(anychart.maps['world']); // title the map map.title("Silk Road Trade Route from Xian to Venice"); // set the container id map.container('container'); // draw the map map.draw(); }); </script> </body></html>
Customizing a JavaScript Connector Map
The initial, basic connector map already gives a general idea of how that Silk Road route looked. But there is something we can quickly change to make it better.
A. Scale the map
B. Add city labels
C. Customize the markers
D. Enhance the tooltip
E. Customize the connectors
F. Add zoom actions and control
FOR A WALKTHROUGH OF THESE JS CONNECTOR MAP CUSTOMIZATIONS, CONTINUE READING HERE.
Original Link: https://dev.to/andreykh1985/how-to-build-a-connector-map-in-js-4b6m

Dev To
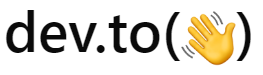
More About this Source Visit Dev To