An Interest In:
Web News this Week
- April 27, 2024
- April 26, 2024
- April 25, 2024
- April 24, 2024
- April 23, 2024
- April 22, 2024
- April 21, 2024
Short-circuiting in JavaScript
Ever heard of short-circuiting in JavaScript? It's not something strange, believe me. You might have even interacted with some parts of it at one point. Well, short-circuiting is a way of simplifying conditionals while making code more readable. In most cases, short-circuiting is utilized in place of if-else expressions. It can be used for both single and multiple if-else statements.
There are various operators used in short-circuiting. They include OR and AND. OR which is denoted by || is used to test a single condition. If one condition is right, then the code executes successfully. Check out the example below;
const response = "Foo";
const username = response || "guest";
console.log(username);
The code above checks if there is a response. If there is one, it returns the response. If not, it returns guest as the username.
AND which is denoted by && is used to test for multiple conditions. For the code to run, all of the requirements must be met. Look at the following illustration;
const response = "Foo";
const isEmailVerified = true;
const username = isEmailVerified && response ;
console.log(username);
This code evaluates when there is a response and the email is verified.
Both AND and OR operators can be used in one operation. For example;
const response = "Foo";
const isEmailVerified = true;
const username = isEmailVerified && response || "guest";
console.log(username);
In such a scenario, the AND is executed before the OR.
There is a technique to 'override' this and allow the OR to run first. This is accomplished by the use of parenthesis. Which operators are run first are specified by parentheses. Here is an example;
const response = "Foo";
const isEmailVerified = true;
const username = isEmailVerified && (response || "guest");
console.log(username);
In this case, the OR is executed before the AND operator.
In conclusion, short-circuiting should not be used as a way to shorten code but as a way to make code more readable.
Original Link: https://dev.to/wangari/short-circuiting-in-javascript-4o2a

Dev To
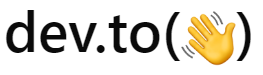
More About this Source Visit Dev To