An Interest In:
Web News this Week
- April 27, 2024
- April 26, 2024
- April 25, 2024
- April 24, 2024
- April 23, 2024
- April 22, 2024
- April 21, 2024
April 12, 2022 06:45 am GMT
Original Link: https://dev.to/ndrohith/creating-an-axios-instance-5b4n
Creating an Axios Instance
In this blog, I'll show you how to create an axios instance in a react project.
I had covered the fundamentals of Axios in my earlier blog.
Once your react app is created lets create a components
folder inside our src
directory .Later, inside our components
folder, create two folders named api
and main
to hold our api instance file and other webpage files.
src|--components |-api |-main
Inside api
directory lets create a file named api_instance.js
. Here i am using my localhost as baseURL.
import axios from "axios"; const instance = axios.create({ baseURL : 'http://127.0.0.1:8000/api/', headers: {// Authorization: `<Your Auth Token>`, Content-Type: "application/json", timeout : 1000, }, // .. other options});export default instance;
After we've finished creating our instance file, we can import it into our js file.
Let's create a file named home.js
inside main
folder
import React, { Component } from "react";import instance from "../api/api_instance";class Home extends Component { constructor(props) { super(props); this.state = {}; } async componentDidMount() { try { await instance({ // url of the api endpoint (can be changed) url: "home/", method: "GET", }).then((res) => { // handle success console.log(res); }); } catch (e) { // handle error console.error(e); } } postData = async (e) => { e.preventDefault(); var data = { id: 1, name: "rohith", }; try { await instance({ // url of the api endpoint (can be changed) url: "profile-create/", method: "POST", data: data, }).then((res) => { // handle success console.log(res); }); } catch (e) { // handle error console.error(e); } }; putData = async (e) => { e.preventDefault(); var data = { id: 1, name: "ndrohith", }; try { await instance({ // url of the api endpoint (can be changed) url: "profile-update/", method: "PUT", data: data, }).then((res) => { // handle success console.log(res); }); } catch (e) { // handle error console.error(e); } }; deleteData = async (e) => { e.preventDefault(); var data = { id: 1, }; try { await instance({ // url of the api endpoint (can be changed) url: "profile-delete/", method: "DELETE", data: data, }).then((res) => { // handle success console.log(res); }); } catch (e) { // handle error console.error(e); } }; render() { return <>Home Page</>; }}export default Home;
That's about all . Your axios instance has been created and can be configured according to your project.
Original Link: https://dev.to/ndrohith/creating-an-axios-instance-5b4n
Share this article:
Tweet
View Full Article

Dev To
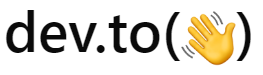
More About this Source Visit Dev To