An Interest In:
Web News this Week
- April 27, 2024
- April 26, 2024
- April 25, 2024
- April 24, 2024
- April 23, 2024
- April 22, 2024
- April 21, 2024
Control program flow with if statement in shell scripting
In the last post, we learned about tests in shell scripting.

Variables and Tests in shell scripting
Twinkle lahariya Apr 6 4 min read
In this post, let's see how we can use them with if statement to control the flow of the script.
What is if
statement?
If statement allows us to make decisions in our script. It starts with the keyword if
followed by a test expression, then
list of commands followed by the closing fi
keyword.
A basic if
statement says that if the test expression is true, then perform the following commands.
Syntax
if []then command 1 command 2 . . command nfi
Let's understand if
with an example where we check if a given number is greater than 10.
#!/bin/bashVAR=20if [ $VAR -gt 10 ]then echo "Variable is greater than 10"fi
Output:
> ./IfDemo.shVariable is greater than 10
Let's see what we have done here:
if [ $VAR -gt 10 ]
: if
followed by a test condition $VAR -gt 10
$VAR -gt 10
: returns true if the var is greater (-gt
) than 10then
: if the condition is true, then execute the following commandsfi
: end of if
statement
What if you want to log both the scenarios, i.e. if the number is greater than 10 and then the number is less than 10.
If..else statement
If-else statement follows the following form:
Syntax
if []then command 1else command 2fi
If-else tells that if the test is true, then run command 1, else run command 2.
Let's extend the same example of a number greater than 10 and print a message if the number is not.
#!/bin/bashVAR=5if [ $VAR -gt 10 ]then echo "Variable is greater than 10"else echo "Variable is less than 10"fi
Output:
> ./IfDemo.shVariable is less than 10
Now, if we want to check the second test condition if the first one fails and handle it differently, then we use if-elif-else
ladder.
If..elif..else statement
Let's check if the number is greater than 10, then print 'greater than'. Else, if the number is equals 10, print 'equals to', else print 'less than'.
#!/bin/bashVAR=5if [ $VAR -gt 10 ]then echo "Variable is greater than 10"elif [ $VAR -eq 10] echo "Variable is equals to 10"else echo "Variable is less than 10"fi
Output:
> ./IfDemo.shVariable is equals to 10
There is more to if statements. To list some of the scenarios:
1: Nested if statements:
if [[ Test condition ]]then if [[ Test condition ]] then command 1 else command 2 fifi
2: Multiple test conditions
if [[ Test condition ]] && [[ Test condition ]]then command 1elif [[ Test condition ]] || [[ Test condition ]]then command 2else command 3fi
Cool, I'll go now and try these scenarios out, do let me know if you try them and have any questions about it.
Up next is loops in shell scripting. Stay tuned.
Until next time. Love
Original Link: https://dev.to/twinklelahariya/control-program-flow-with-if-statement-in-shell-scripting-1b91

Dev To
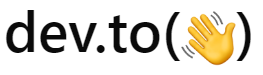
More About this Source Visit Dev To