An Interest In:
Web News this Week
- April 27, 2024
- April 26, 2024
- April 25, 2024
- April 24, 2024
- April 23, 2024
- April 22, 2024
- April 21, 2024
April 7, 2022 12:42 am GMT
Original Link: https://dev.to/gregorygaines/how-to-properly-hash-and-salt-passwords-in-golang-using-bcrypt-io4
How to Properly Hash and Salt Passwords in Golang Using Bcrypt
Prerequisites
- Download the golang bcrypt library using
go get golang.org/x/crypto/bcrypt
.
Hashing
// Hash password using the bcrypt hashing algorithmfunc hashPassword(password string) (string, error) { // Convert password string to byte slice var passwordBytes = []byte(password) // Hash password with bcrypt's min cost hashedPasswordBytes, err := bcrypt. GenerateFromPassword(passwordBytes, bcrypt.MinCost) return string(hashedPasswordBytes), err}func main() { // Hash password var hashedPassword, err = hashPassword("password1") if err != nil { println(fmt.Println("Error hashing password")) return } fmt.Println("Password Hash:", hashedPassword)}
No Need to Salt Passwords
Bcrypt uses a concept named cost
which represents the number of hash iterations that bcrypt undertakes. Hashing time is calculated as 2 ^ cost
and the higher the cost, the longer the hashing process takes.
This deters attackers because they can't quickly brute force a password match and increasing computational power will do little to help. Bcrypt uses a variable named bcrypt.MinCost
as a default cost and any cost lower defaults to bcrypt.DefaultCost
.
Password Matching
package mainimport ( "fmt" "golang.org/x/crypto/bcrypt")// Hash passwordfunc hashPassword(password string) (string, error) { // Convert password string to byte slice var passwordBytes = []byte(password) // Hash password with Bcrypt's min cost hashedPasswordBytes, err := bcrypt. GenerateFromPassword(passwordBytes, bcrypt.MinCost) return string(hashedPasswordBytes), err}// Check if two passwords match using Bcrypt's CompareHashAndPassword// which return nil on success and an error on failure.func doPasswordsMatch(hashedPassword, currPassword string) bool { err := bcrypt.CompareHashAndPassword( []byte(hashedPassword), []byte(currPassword)) return err == nil}func main() { // Hash password var hashedPassword, err = hashPassword("password1") if err != nil { println(fmt.Println("Error hashing password")) return } fmt.Println("Password Hash:", hashedPassword) // Check if passed password matches the original password fmt.Println("Password Match:", doPasswordsMatch(hashedPassword, "password1"))}
Password Hash: $2a$04$kcsOln10l5Gm0lSgQmlrhuS0T9u124J3HfrAr9tnHltr9u7.iUsJmPassword Match: true
Consider signing up for my newsletter or supporting me if this was helpful. Thanks for reading!
Original Link: https://dev.to/gregorygaines/how-to-properly-hash-and-salt-passwords-in-golang-using-bcrypt-io4
Share this article:
Tweet
View Full Article

Dev To
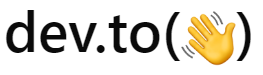
More About this Source Visit Dev To