An Interest In:
Web News this Week
- April 27, 2024
- April 26, 2024
- April 25, 2024
- April 24, 2024
- April 23, 2024
- April 22, 2024
- April 21, 2024
Differences between the factory pattern and the constructor pattern in JavaScript.
Repeating code is something that's avoidable when writing logic for a piece of software that you need to produce.
Abstracting logic is a strategy that can be employed to avoid the repeatition that could emanate.
By the end of this article, you'll have learnt how to;
- Use the factory pattern
- Use the constructor pattern
- The difference between the constructor and factory patterns.
Factory pattern
A factory in programming describes a function that returns an object...
function myFunc() { let obj = {}; return obj;}const newObj = myFunc();
Factories can be used to set properties and methods on the objects that they return. In the code snippet below, the makeAnimal
function is used to create other objects that represent animals. When using factories, property values are assigned to the returned object through the function's arguments.
function makeAnimal(name, legs, horns) { let animal = {}; // Set properties for the animal object animal.name = name; animal.legs = legs; animal.horns = horns; return animal;}const newAnimal = makeAnimal('Cow', 4, 2);console.log(newAnimal.name); // Output: Cowconsole.log(newAnimal.legs); // Output: 4console.log(newAnimal.horns); // Output: 2
Factories can also be used to create default values for properties of the object to be returned.
function makeAnimal(name: string, legs: number, horns: number) { let animal = {}; // Set properties for the animal object animal.name = name; animal.legs = legs; animal.horns = horns; animal.hasFur = true; //Every new object returned from this function will have the `hasFur` property and it's value will always be true unless it's changed. return animal;}tconst newAnimal = makeAnimal('Cow', 4, 2);console.log(newAnimal.name); // Output: Cowconsole.log(newAnimal.horns); // Output: true
Constructor pattern
This is another pattern used to create objects in javascript. Like a factory, a constructor is a function that returns an object.
You could also declare
Refer to the code snippet below.
function Animal(name: string, legs: number, horns: number) { // Set properties for the animal object this.name = name; this.legs = legs; this.horns = true; //Default property}const newAnimal = new Animal('Cow', 4, 2);console.log(newAnimal.name); // Output: Cow
Or, there's a cooler way to declare constructors in Javascript with some syntaxical sugar:
class Animal { constructor(legs) { this.legs = legs; this.printLegs = function () { console.log('Animal has %d legs:', this.legs); }, this.name = 'Animal'; }}let animal = new Animal(4);animal.printLegs();//Output : Animal has 4 legs
Differences between the constructors and factories
Syntax.
Factories do not use thenew
keyword whereas constructors do. Refer to the examples above.Constructors inherit from a prototype property and return
true
frominstanceOf factoryFunction
.
Factories, on the other hand do not inherit a reference to theprototype
property and returnfalse
frominstanceOf factoryFunction
.
Original Link: https://dev.to/luigimorel_1/differences-between-the-factory-pattern-and-the-constructor-pattern-in-javascript-382b

Dev To
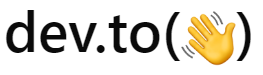
More About this Source Visit Dev To