An Interest In:
Web News this Week
- April 27, 2024
- April 26, 2024
- April 25, 2024
- April 24, 2024
- April 23, 2024
- April 22, 2024
- April 21, 2024
JavaScript Array Methods - Shift, Unshift, Push and Pop
Hey, wa'SUPP everyone, it's me again! I learned how to add emoji in my post, now I need to learn not to overuse them...
Today, I will be talking about 4 different JavaScript (JS) array methods, which I have given the acronym "SUPP". The four different methods are .shift()
, .unshift()
, .push()
and .pop()
.
These four methods are relatively short so you will be able to learn them in no time. All four methods are used to modify the array, either at the beginning or at the end of the array.
NOTE: These methods can be used on array-like objects by using .call()
or .apply()
. For information on what is the difference between an Array and an Array-like Object () check out this Stack Overflow Question.
Array.prototype.shift()
The .shift()
method removes and returns the first element in the array, i.e. the zeroth index element. This method changes the array, and the length will be shorten by...1. If you want to do something with the shifted element, you can assign a variable to it.
Array.prototype.unshift()
The .unshift()
method allow you to add elements at the beginning of the array and it returns the new length of the array to the caller.
Example -
const rainbowColors = ["red", "orange", "yellow", "green", "blue", "indigo", "violet"];// Shift Exampleconst shiftedElement = rainbowColors.shift();console.log(shiftedElement + " was removed"); // "red was removed"console.log(rainbowColors); // ["orange", "yellow", "green", "blue", "indigo", "violet"]// Unshift Exampleconst newRed = "bright red";const newLength = rainbowColors.unshift(newRed, "light orange");console.log(newLength); // 8console.log(rainbowColors); // ["bright red","light orange","orange", "yellow", "green", "blue", "indigo", "violet"]
Array.prototype.pop()
The .pop()
method removes and returns the element at the end of the array. To use the removed element later, just store it as a variable.
Array.prototype.push()
The .push()
method allow you to add elements at the end of the array and it returns the new length of the array.
Example - (I will show you the example on an Array-like Object)
// function that returns argumentsfunction getArguments() { return arguments;}const passedArguments = getArguments("one", "two", "three", "four" );// calling .pop() on an Array-like Objectconst poppedElement = Array.prototype.pop.call(passedArguments)console.log(poppedElement); // "four"// calling .push() on an Array-like Objectconsole.log(Array.prototype.push.call(passedArguments, "five", "six")); // 5
In JS, there is a built-in arguments
object which is an Array-like Object containing the arguments that were passed into the function. We cannot use array methods on the objects directly, so we have to use .call()
and pass the Array-like Object as the first argument, just as we did in the example above. Alternatively, we could create an array from the Array-like Object and call the array method directly... I think I will do a lesson on creating/change the array next.
Summary
SUPP are very useful when you want to add/remove elements to the beginning or end of an Array (or Array-like Objects), so be sure to practice with them when you are learning.
Remember, you can always look at the documentation for greater details and more examples at MDN Web Doc
Thank you for reading, if you have any feedback/comments please let me know.
Original Link: https://dev.to/justtanwa/javascript-array-methods-shift-unshift-push-and-pop-1fgi

Dev To
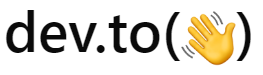
More About this Source Visit Dev To