An Interest In:
Web News this Week
- April 27, 2024
- April 26, 2024
- April 25, 2024
- April 24, 2024
- April 23, 2024
- April 22, 2024
- April 21, 2024
useEffect vs useLayoutEffect: the difference and when to use them
I sort of noticed that alot of people do not know the difference between the useEffect
and useLayoutEffect
hooks in React. Finding a use case for useLayoutEffect
seems like a very hard task.
Let's dive straight into it and see what this hooks are really all about, their difference(s) and when to use them.
useEffect
useEffect
allows you perform side effects from a function component. When useEffect is called, React knows to render your side effect only after changes are made to the DOM
By default, React runs the effect after every render - including the first render. This is to say that useEffect
is effected only after the component is rendered.
But if you need your side effect to fire synchronously after the DOM mutation, that is, before the next browser paint without the user ever noticing any flickering or visual inconsistency then you need to use useLayoutEffect
useLayoutEffect
useLayoutEffect
fires synchronously after the DOM mutation and before the browser get to paint the new changes. This hook is especially useful for performing DOM measurement like scroll height, scroll width, scroll position and other style on any DOM element.
Let's make some example codes
useEffect(()=>{ console.log("I will run second instead of first")});useLayoutEffect(()=>{ console.log("I will run first")})
Javascript script is a single-threaded programming language. Suffice to say, it runs from top to bottom. But here if you check the console, "I will run first", that is, the useLayoutEffect
actually runs before the useEffect
runs. This is because useLayoutEffect
is fired synchronously after DOM is mutated and before the browser paints the new changes.
Okay, I will like you to notice the change well in order to better understand how it works. Let's reference a DOM element with the useRef
React hook and let's perform some changes with the two side effect hooks we've been talking about
import React, {useRef, useEffect, useLayoutEffect) from 'react'const App = () => { const inputRef = useRef(null) useEffect(()=>{ inputRef.current.value = "another user" }); useLayoutEffect(()=>{ console.log(inputRef.current.value) }); return( <div> <input type="text" value="EmmanuelTheCoder" ref= {inputRef}/> </div> );}export default App;
Once the component is rendered, the input value becomes "another user" but the console says "EmmanuelTheCoder".
The useLayoutEffect already fired synchronously after the DOM mutation, before the browser could paint "another user"
I hope you got it.
Hey wait, one more thing!
React 18 brought some really good update to useEffect
hook.
Starting in React 18, the function passed to useEffect will fire synchronously before layout and paint when its the result of a discrete user input such as a click, or when its the result of an update wrapped in https://reactjs.org/docs/react-dom.html#flushsync. This behavior allows the result of the effect to be observed by the event system, or by the caller of https://reactjs.org/docs/react-dom.html#flushsync.
Incase you don't know about React 18, I will put out an article on this blog shortly explaining it in detail, plus the newly added hooks.
Thanks for reading.
Original Link: https://dev.to/emmanuelthecoder/useeffect-vs-uselayouteffect-the-difference-and-when-to-use-them-124c

Dev To
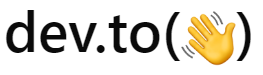
More About this Source Visit Dev To