An Interest In:
Web News this Week
- April 18, 2024
- April 17, 2024
- April 16, 2024
- April 15, 2024
- April 14, 2024
- April 13, 2024
- April 12, 2024
JavaScript Array Methods - Some & Every
Today, I will tell you about .some()
and .every()
. Double knowledge. I don't use these as often as .map()
or .filter()
but they do come in quite handy in certain scenarios. I have grouped these methods together because they both return a Boolean value as a result, so it made sense (in my mind) to learn them together. Plus they are very short.
Array.prototype.some()
Just like the other methods I have talked about so far, .some()
also take a function as its argument (a.k.a callback). The .some()
method returns true
for the first value/element in the array which passes the test and false
otherwise.
I will use the typeof
operator in my example, this operator returns a string telling you what the data type is.
Example:
let stoppingIndex;const arrOfDataTypes = ["this is a string", 93, false, 72n, true, false, { name: "Tanwa", hobby: ["coding", "gaming"] }]function callbackCheckBool(a) { return typeof a === 'boolean';}console.log(arrOfDataTypes.some( (element, index) => { stoppingIndex = index; return callbackCheckBool(element); }));// trueconsole.log(stoppingIndex); // 2console.log(arrOfDataTypes.some( element => typeof element === 'undefined'));// false
In the above example, we wanted to check if there are some value/element in the array that are of the data type 'boolean'
, this return true
because the array contains the element "false"
which is a Boolean type at index 2
where the .some()
method stopped. In the second .some()
test, we check for data type of 'undefined'
, as the array does not have this type of value it returned false
.
Array.prototype.every()
If you guess that .every()
method returns true
only when every value/element in the array passes the callback function test, then you would be correct! I think of .every()
as the opposite to .some()
as it will return the false
as soon as one element fails the test.
Example:
const arrOfStrings = ["How", "long", "is", "a", "piece", "of", "string?", 1];const arrOfTwos = [2,2,2,2,2];console.log(arrOfStrings.every( element => typeof element === 'string')); // falseconsole.log(arrOfTwos.every( element => element === 2)); // true
I hope the example above is straight forward, we checked if every value/element in the array is of the type 'string'
but this return false
because of the sneaky number 1
at the end! And in the second .every()
test, it returns true
as all the element passed the test.
Summary
The .some()
method checks for first value/element in the array that passes the callback function test and returns true
and if it does not find any that passes the test it returns false
. While the .every()
method checks for the first value/element in the array that fails the callback function test and returns false
or it returns true
if all the value passes the test.
If you made it this far, thank you for reading, and as always please leave comment and feedback if I have misunderstood anything.
Original Link: https://dev.to/justtanwa/javascript-array-methods-some-every-30bb

Dev To
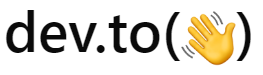
More About this Source Visit Dev To