An Interest In:
Web News this Week
- April 2, 2024
- April 1, 2024
- March 31, 2024
- March 30, 2024
- March 29, 2024
- March 28, 2024
- March 27, 2024
Cookie Banners
You've probably seen the pop-up block at the bottom of various sites.
It looks something like this:
Let's layout something like that =)
HTML
<div class="warning warning--active"> <div class="warning__text">This website uses cookies.</div> <button class="warning__apply" type="button">OK</button> <a class="warning__read" href="https://ru.wikipedia.org/wiki/Cookie">Read more</a></div>
Our banner will consist of text, a confirmation button, and a link to an explanatory document.
Basic CSS
.warning { position: fixed; bottom: 0; left: 0; display: none; width: 100%;}.warning--active { display: flex;}
Position the die in the lower left corner and stretch it across the width.
JavaScript
In our script, we need to:
- Make sure the block is there - otherwise stop working;
- Find the block and confirm button;
- Hover over the "listener" button;
- When the button is clicked, remove the
warning--active
class from the block, thereby hiding it; - Save a cookie that will tell us that the user clicked the button.
let container;let apply;const init = () => { if (!who()) { // stop the script return; } findElements(); subscribe();};
Who
If there is no block on the page - the function will return false:
const who = () => document.querySelector(".warning");
findElements()
Find the block itself and the button inside:
const findElements = () => { container = document.querySelector(".warning"); apply = container.querySelector(".warning__apply");};
subscribe()
Add a "click" event handler to the button:
const subscribe = () => { apply.addEventListener("click", onClick);};
onClick()
const onClick = (event) => { // Override the default behavior event.preventDefault(); // Hide the block hideContainer(); // Set cookies setCookie();};
hideContainer()
const hideContainer = () => { container.classList.remove("warning--active");};
In the main css section, you can see that the warning
class has the display: none;
property, and the warning--active
class has the display: flex;
property.
By removing warning--active
we hide the block.
setCookie()
const setCookie = () => { document.cookie = "warning=true; max-age=2592000; path=/";};
Let's set a cookie that will tell us that the user has agreed to the use of cookies.
2592000 - number of seconds in a month
Once the cookie is set, it is assumed that the block will no longer appear on the page.
This can be achieved in two ways
Through the back
Please ask the backend or yourself (if you are the backend) not to display the block if the user has the cookies you set.
The author of this article prefers the backend method =)
Through the front
Let's rewrite the who()
function, which will look for cookies instead of checking for a block:
const who = () => { // If there are cookies, it will return true return getCookie('warning');};
The implementation of the
getCookie
function can be seen at learn.javascript.ru
Remove the warning--active
class from the HTML
<div class="warning warning--active">
By default, the block will be hidden. Let's show it if the script didn't find the cookie:
const showContainer = () => { container.classList.add("warning--active");};const init = () => { if (!who()) { return; } findElements(); showContainer(); subscribe();};
Everything else is unchanged.
Full script:
let container;let apply;const who = () => document.querySelector(".warning");const findElements = () => { container = document.querySelector(".warning"); apply = container.querySelector(".warning__apply");};const hideContainer = () => { container.classList.remove("warning--active");};const setCookie = () => { document.cookie = "warning=true; max-age=2592000; path=/";};const onClick = (event) => { event.preventDefault(); hideContainer(); setCookie();};const subscribe = () => { apply.addEventListener("click", onClick);};const init = () => { if (!who()) { return; } findElements(); subscribe();};init();
Or on codepen
The full script can be reduced to 8 lines
if (document.querySelector(".warning")) { const container = document.querySelector(".warning"); const apply = container.querySelector(".warning__apply"); apply.addEventListener("click", () => { container.classList.remove("warning--active"); document.cookie = "warning=true; max-age=2592000; path=/"; });};
Original Link: https://dev.to/vadimfilimonov/cookie-banners-4nb4

Dev To
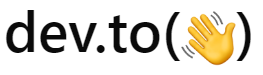
More About this Source Visit Dev To