An Interest In:
Web News this Week
- April 26, 2024
- April 25, 2024
- April 24, 2024
- April 23, 2024
- April 22, 2024
- April 21, 2024
- April 20, 2024
NumPy Basics : Part 2
This article is a continuation of my previous post on NumPy here.
NumPy Operations
1.Adding an element to an array
Use numpy.append() to add to the end of the array.
array1 = np.append(array_x, 1)array1
Output:array([ 5, 6, 7, 8, 9, 1])
Note: A numpy array does not support append() method directly.
2.Removing an element in the array
Use numpy.delete() to remove the element at a particular index.
array2 = np.delete(array1,2)array2
Output:array([ 5, 6, 8, 9, 1])
3.Sorting an array.
array3 = np.sort(array2)array3
Output:array([1, 5, 6, 8, 9])
4.Reshaping an array
Use np.reshape(). The array you intend to output must have the same number of elements.
For instance if an 3x4 array has 12 elements, then you can reshape it to 6x2 or 4x3 or 2x6.
You can use numpy.reshape() directly on a numpy array.
arr2 = np.reshape(array_A,(3,2))arr2
Output:array([[2, 4],[6, 1],[3, 5]])
5.Flattening an array
Use flatten() to return a one dimension array.
arr2.flatten()
Output:array([2, 4, 6, 1, 3, 5])
You can also use ravel() to flatten the array.
array_A.ravel()
Output:array([2, 4, 6, 1, 3, 5])
Note: reshape(), flatten(),ravel() does not affect the original array.
NumPy Arithmetic Operations
In this section, we will cover specific functions in NumPy used in arithmetic operations.
**Note: **You can only perform arithmetic operations if the arrays have the same structure and dimensions.
1.Add
Addition can be done using np.add() or using the + arithmetic operator. The output is an ndarray object.
import numpy as npa = np.array([20,30,40,50,60])b = np.array([4,5,6,7,8])np.add(a, b)
Output:array([24, 35, 46, 57, 68])
c = a+bprint(c)print(type(c))
Output:
[24 35 46 57 68]
2.Subtract
Subtraction can be done using np.subtract() or using the -arithmetic operator.
import numpy as npa = np.array([20,3,40,50,60])b = np.array([4,5,6,7,8])c = a-bprint(c)
Output: [16 -2 34 43 52]
Using np.subtract() the second argument is subtracted from the first.
import numpy as npa = np.array([20,30,40,50,60])b = np.array([4,5,6,7,8])np.subtract(b, a)
Output:array([-16, -25, -34, -43, -52])
3.Multiply
Multiplication can be done using np.multiply() or using the * arithmetic operator.
import numpy as npa = np.array([20,30,40,50,60])b = np.array([4,5,6,7,8])print(np.multiply(b, a))print(a*b)
Output:[ 80 150 240 350 480]
[ 80 150 240 350 480]
4.Divide
Division can be done using np.divide() or using the / arithmetic operator.
import numpy as npa = np.array([20,30,40,50,60])b = np.array([4,5,6,7,8])print(np.divide(b, a))print(a/b)
Output:
[5. 6. 6.66666667 7.14285714 7.5]
[5. 6. 6.66666667 7.14285714 7.5]
5.Statistical Functions
You can get the sum,mean, average and variance of all the elements in an array.
import numpy as npa = np.array([2.5,3.5 ,4.3,5.4,6.5])print('The sum is ', np.sum(a))print('The mean is ', np.mean(a))print('The average is ', np.average(a))print('The variance is ', np.var(a))
Output:
The sum is 22.200000000000003The mean is 4.44The average is 4.44The variance is 1.9664000000000001
6.Power function
The power() function performs the power of two arrays where the first argument is the base raised to the power of the second argument.
import numpy as npa = np.array([2,3,4,5,6])b = np.array([5,4,3,2,1])print(np.power(a, b))
Output:
[32 81 64 25 6]
7.Remainder and Modulus
The remainder() function gives the remainder of the two arrays similar to the mod() function.
import numpy as npa = np.array([2,3,4,5,6])b = np.array([5,4,3,2,1])print(np.mod(a, b))print(np.remainder(a,b))
Output:
[2 3 1 1 0][2 3 1 1 0]
8.Reciprocal
The reciprocal() function returns the reciprocal of each element in the array.
import numpy as npa = np.array([2.5,3.5 ,4.3,5.4,6.5])print(np.reciprocal(a))
Output:array([ 1., 3.25, 5.5 , 7.75, 10.])
9.Minimum and Maximum
You can get the minimum using min() function and maximum using max() function.
array_x = np.array([5,6,7,8,9])print('The max is' ,array_x.max())print('The min is' ,array_x.min())
Output:
The max is 9The min is 5
This is wraps up the summary of some of the most popularly used NumPy operations.
Original Link: https://dev.to/wanguiwaweru/numpy-basics-part-2-o5k

Dev To
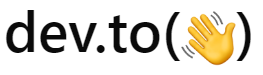
More About this Source Visit Dev To