An Interest In:
Web News this Week
- April 19, 2024
- April 18, 2024
- April 17, 2024
- April 16, 2024
- April 15, 2024
- April 14, 2024
- April 13, 2024
January 23, 2022 06:08 pm GMT
Original Link: https://dev.to/buddhadebchhetri/javascript-es6-ilj
JavaScript ES6
JavaScript was invented by Brendan Eich in 1995, and became an ECMA standard in 1997.
ECMAScript is the official name of the language.
ECMAScript versions have been abbreviated to ES1, ES2, ES3, ES5, and ES6.
Since 2016 new versions are named by year (ECMAScript 2016 / 2017 / 2018).
Arrow Function
const sum =(a,b)=> a+bconsole.log(sum(2,6)//prints 8
Default parameters
function print(a=5){console.log(a)}print();//prints 5
Let Scope
let a=3;if(true){let a=5;console.log(a);//prints 5}console.log(a);//prints 3
Const
//can be assigned only oncevar x = 50;// Here x is 50{ const x = 16; console.log(x);// Here x is 16}console.log(x); // Here x is 50
Multiline String
console.log('This is a multiline string');
Template string
const name ='World'const message ='Hello ${name}'console.log(message)//Prints Hello World
Exponent Operator
const byte =2**8//same as : Math.pow(2,8)
Spread Operator
Const a=[1,2]const b=[3,4]const c=[...a,...b]console.log(c) //[1,2,3,4]
String Includes()
Console.log('apple'.includes('p1))//Prints trueconsole.log('apple'.includes('tt))//prints false
String StartsWith()
console.log('ab.repeat(3))//prints 'ababab'
Destructuring array
let [a,b] =[3,7];console.log(a);//3console.log(b);//7
Destucturing object
let obj ={a:55,b:44};let{a,b}= obj;console.log(a);//55console.log(b);//44
Object property assignment
const a=2const b=5const obj={a,b}//before es6://obj ={a:a,b:b}console.log(obj3);//{a:2,b:5}
Object.Assign()
const obj1 ={a:1}const obj2 ={b:2}const obj3 =object.assign({},obj1,obj2)console.log(obj3)//{a:1 ,b:2}
Promises with finally
promise.then ((result) => {...}).catch(error) => {..}).finally(() => { //Logic independent of success/error })
Original Link: https://dev.to/buddhadebchhetri/javascript-es6-ilj
Share this article:
Tweet
View Full Article

Dev To
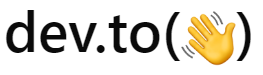
More About this Source Visit Dev To