An Interest In:
Web News this Week
- April 2, 2024
- April 1, 2024
- March 31, 2024
- March 30, 2024
- March 29, 2024
- March 28, 2024
- March 27, 2024
How to Create a Button in JavaScript
Learn how to create a button in JavaScript and add it to your HTML page.
As a web developer, you should probably create buttons and add them to your HTML page programmatically.
A typical workflow is to validate some data in your JavaScript code before displaying a button in HTML to your users.
Before starting, let me show you what you will achieve!
<button type="button">Can you click me?</button>
When you click on the button, your JavaScript code will trigger an alert.
Let's dive into that tutorial!
1. Create a button using document createElement
To create a button in JavaScript, you must use the createElement
function available by default in the Document interface of your web page.
Then, you will configure the button with a "Can you click me?" text.
// Create a button elementconst button = document.createElement('button')// Set the button text to 'Can you click me?'button.innerText = 'Can you click me?'
As a side note, you can add a lot of properties to a button when creating it. This tutorial only sets the innerText
because we don't need the other properties.
2. Add an event listener on your button
The next step is to create an event listener on your button. It will allow you to detect when a user clicks on it.
Whenever the "click" event is detected, the arrow function (second parameter) is launched.
// Attach the "click" event to your buttonbutton.addEventListener('click', () => { // When there is a "click" // it shows an alert in the browser alert('Oh, you clicked me!')})
3. Add your JS button to your HTML
The last step is to add your button to the HTML body.
After that, you will have the same result as the beginning example!
// Add the button to your HTML <body> tagdocument.body.appendChild(button)
Full code to create a button in JS
<html> <head> <title>Create a Button in JavaScript</title> </head> <body> <!-- Empty <body> HTML (the JavaScript code in <script> will generate a button here)--> <script> // Create a button element const button = document.createElement('button') // Set the button text to 'Can you click me?' button.innerText = 'Can you click me?' button.id = 'mainButton' // Attach the "click" event to your button button.addEventListener('click', () => { // When there is a "click" // it shows an alert in the browser alert('Oh, you clicked me!') }) document.body.appendChild(button) </script> </body></html>
What's next?
Congrats! Now you know how to programmatically create buttons using JavaScript. You can go a bit further by learning how to enable and disable a button in JavaScript.
I'm starting to tweet more consistently. If you want to get more tips and resources about web development, developer tips, and my journey as a Full-stack Engineer -> Find me on my Twitter
Original Link: https://dev.to/gaelgthomas/how-to-create-a-button-in-javascript-2cf

Dev To
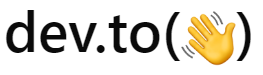
More About this Source Visit Dev To