An Interest In:
Web News this Week
- April 1, 2024
- March 31, 2024
- March 30, 2024
- March 29, 2024
- March 28, 2024
- March 27, 2024
- March 26, 2024
React Conditional rendering
In this post we render an element on condition.
To show and to hide an element by click.
Create a sample react-app.
Open console, type npx create-react-app and application name.
Open the application folder and type npm start to run the web-server.
Open the App.js file and remove all the content.
Import react.
Add an element to render on condition. Let it be a toolbar placeholder.
In the App-component set state to remember if the element has to be rendered.
Define a method to toggle the state from visible to hidden.
In the render method there are two elements: a button to toggle the state and a toolbar to render on condition.
The toolbar is in curly braces. Its an expression with a logical AND-operator.
First operand is the condition. Second operand is the element to render.
If the condition is true the expression evaluates to the element. The element is rendered.
When the condition is false the expression evaluates to false. The element is not rendered.
Lets check it out.
Initially the toolbar is not visible.
Click on the button to toggle the state.
The element is rendered.
The element is added to the document and is removed from the document.
import React from "react";function Toolbar() { return (<div>Toolbar component placeholder</div>);}class App extends React.Component { constructor(props) { super(props); this.state = {editMode: false} } toggle = () => { this.setState({editMode: !this.state.editMode}); } render() { return ( <> <button onClick={this.toggle}>{this.state.editMode ? 'To View' : 'To Edit'}</button> {this.state.editMode && <Toolbar/>} </> ); }}export default App;
Video-tutorial: https://youtu.be/MyCBexcqHZI
See you next time. Have a nice day!
Original Link: https://dev.to/101samovar/react-conditional-rendering-5a44

Dev To
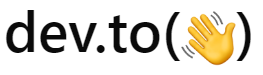
More About this Source Visit Dev To